Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial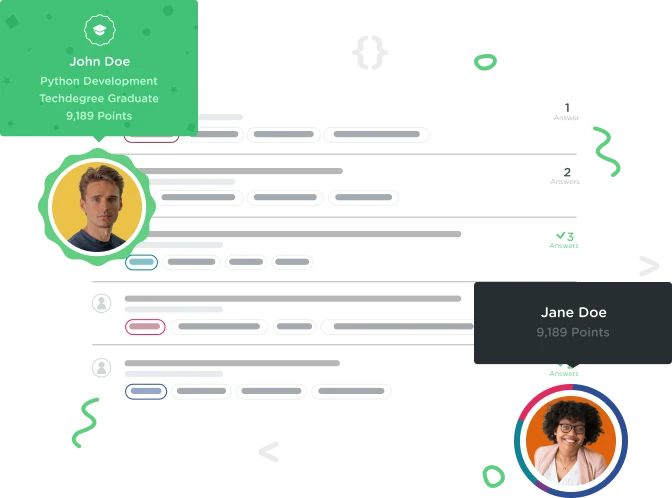

Jason Ladieu
10,635 PointsLinked list recursion processing
Recursion is going to be the death of me... All kidding aside, is there a kind soul out there who can help me understand how this recursive method is processing a linked list of integers? I do not understand the logic, and I think is it mainly the recursion that is throwing me off. I fear I need some detailed insight into the logic happening here.
public class IntNode {
int item; // One of the integers in the list.
IntNode next; // Pointer to the next node in the list.
}
/**
* Compute the sum of all the integers in a linked list of integers.
* @param head a pointer to the first node in the linked list
*/
public static int addItemsInList( IntNode head ) {
if ( head == null ) {
// Base case: The list is empty, so the sum is zero.
return 0;
} else {
// Recursive case: The list is non-empty. Find the sum of
// the tail list, and add that to the item in the head node.
// (Note that this case could be written simply as
// return head.item + addItemsInList( head.next );)
int listsum = head.item;
int tailsum = addItemsInList( head.next );
listsum = listsum + tailsum;
return listsum;
}
}