Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial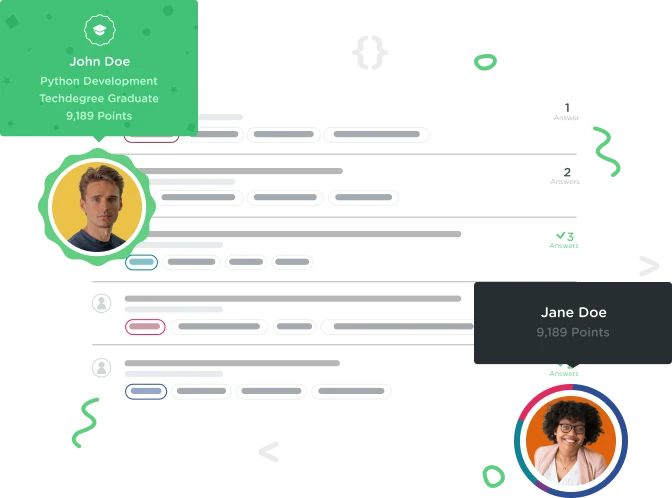

Callum Lynch
5,972 PointsLinking a random string generator to its correct Image
I have successfully created a random string generator that works perfectly in my app. The problem is that I want the correct image, linked to the randomly selected string to be shown below it. How do I use the result of the random string generator to then look through a different Array and then display the correct image? Or is there a different way of doing what i'm trying to do?
2 Answers
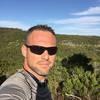
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsWith the way you currently have it set up, you can take the methods out of your structs. Unless your team lists are going to be huge you could also just not use structs all together. I haven't tested the code below but it looks like it should work.
struct TeamNameProvider {
let teamName = ["teamOne", "teamTwo", "teamThree", "teamFour"]
}
struct TeamBadgeProvider {
let teamBadge = [badgeOneImage, badgeTwoImage, badgeThreeImage, badgeFourImage]
}
var currentSelectedTeam = ""
var currentSelectedBadge: UIImage = defaultImage
func randomName() {
let teamNameProvider = TeamNameProvider()
let teamBadgeProvider = TeamBadgeProvider()
let randomNumber = GKRandomSource.sharedRandom().nextInt(upperBound: teamNameProvider.teamName.count)
currentSelectedTeam = teamNameProvider.teamName[randomNumber]
switch currentSelectedTeam {
case "teamOne":
currentSelectedBadge = teamBadgeProvider.teamBadge[0]
case "teamTwo":
currentSelectedBadge = teamBadgeProvider.teamBadge[1]
case "teamThree":
currentSelectedBadge = teamBadgeProvider.teamBadge[2]
case "teamFour":
currentSelectedBadge = teamBadgeProvider.teamBadge[3]
default:
currentSelectedBadge = defaultImage
}
}
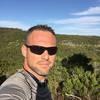
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsAlso instead of sharing an image link you can just copy and paste your code in the following format.
```Swift
//Your code here
``` //Followed by 3 back ticks

Callum Lynch
5,972 PointsThank you for taking the time to help me! One thing that I should have mentioned is that im trying to get the team name to appear in a Label and the corresponding Badge to appear in an Image View. I've tried to play around with the code you offered above but whenever i click my Generate Team Button...it seems to be focusing on the var currentSelectedTeam and var currentSelectedBadge lines. This means that when pushing to my Image View and Label, its simply returning an empty string and my default image, rather than using the randomly selected team and its corresponding badge.
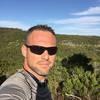
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsDo you have a Git repository for this?
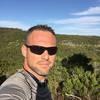
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsHave you thought about using a dictionary or array of dictionaries (depending on your needs). Are the name and image all you need? In that case the key would be the name and the value the image. Or if you need more details like the team stadium, history of the team, sponsors etc than use an array of dictionaries. Another would be to use a plist. Especially if you are going to populate a tableview I would think about using one of these methods. Otherwise keeping it simple instead of returning the team name just set it to a variable and then use a switch statement to return the corresponding image.
Callum Lynch
5,972 PointsCallum Lynch
5,972 Pointshttps://imgur.com/a/g4TOs
This is a link to the code that I currently have.