Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial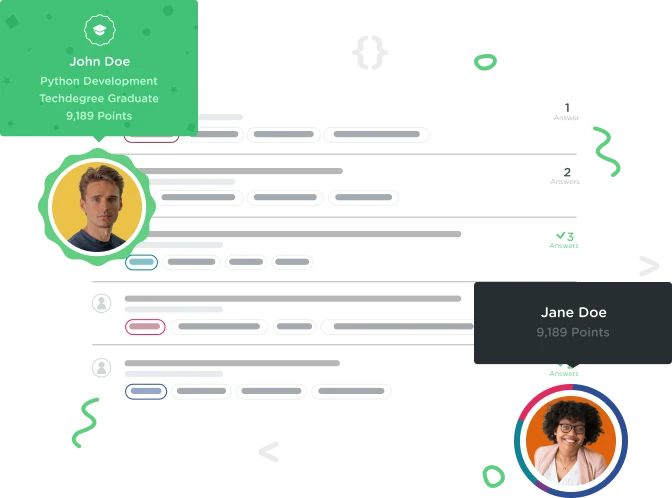
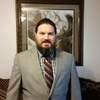
David Batton
10,383 PointsLINQ list of strings in a string
Challenge Task 2 of 2 Now, create a public static method inside the ContainsAnyExtension named ContainsAny that returns a bool - since this is an extension method, the first parameter of the method should be a string preceded by the this keyword, named source. The second parameter should be an IEnumerable<string> named stringsToMatch. Inside the method, use a LINQ query to identify if any of the stringsToMatch are found as partial matches in the source string. Be sure to return true if at least one of the strings in stringsToMatch are found. The search should be case sensitive.
I do not understand how to set this search up. I've been at it a while now and would like to ask for some assistance figuring it out.
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class ContainsAnyExtension
{
public static bool ContainsAny(this string source, IEnumerable<string> stringsToMatch)
{
return stringsToMatch.Any(s => s.Contains(source));
}
}
}
5 Answers
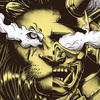
Joshua C
51,696 PointsThe challenge may not be too clear, but it asks you to see if the 'source' contains any of the strings in the 'stringsToMatch' list. In your code, you're checking to see if the 'stringsToMatch' list contains the 'source' input string, which evaluates to false unless the list contains the exact string in 'source'.
When I enter your code in the code challenge I get the error where it says that "Woohoo!" is passed as the source and "Woo, hoo, oo" is passed as the stringsToMatch list. Since stringsToMatch doesn't contain the string "Woohoo!", your method returns false.
Instead your method should return:
stringsToMatch.Any(word => source.Contains(word));
This code checks to see whether 'source' contains any of the strings in 'stringsToMatch', which is what you want.
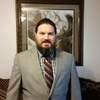
David Batton
10,383 PointsFYI, I did solve it the old fashion way, but I'd like to understand how to do it using a LINQ query.
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class ContainsAnyExtension
{
public static bool ContainsAny(this string source, IEnumerable<string> stringsToMatch)
{
foreach(string word in stringsToMatch)
{
if(source.Contains(word))
{
return true;
}
}
return false;
}
}
}

Kevin Gates
15,053 PointsAfter a lot of researching, I believe this is the simplest answer that is in accordance with what they were expecting:
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class ContainsAnyExtension
{
public static bool ContainsAny(this string source, IEnumerable<string> stringsToMatch)
{
return stringsToMatch.Any( strings => source.Contains(strings));
}
}
}
Essentially the return statement returns a boolean (true / false).
First, the stringsToMatch.Any(...) is looking for anything in it's IEnumerable "List" to return true, and if so, the .Any(...) also returns true to the Return statement -- meaning one of the words passed in by the user was matched with one of our strings.
Second the ** strings => source.Contains(strings) ** is passing in the variable "strings" (which is the parameter data that is an item in the IEnumerable "List"). The function has us check to see if our source parameter contains any of the strings we're checking against. If it's False, it loops to the next item in the IEnumerable "List", else it returns True then it fulfills the Any function, which means, our Contains Any method is returning True also.
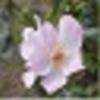
Katrin Nitsche
23,462 PointsI was also struggling with this exercise. This was my solution and it was accepted:
public static bool ContainsAny(this string source, IEnumerable<string> stringsToMatch)
{
bool result = false;
stringsToMatch.ToList().ForEach(s => { if(source.Contains(s)) result = true; });
return result;
}
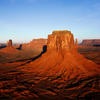
Tim Strand
22,458 PointsThis also works: return (from s in stringsToMatch where source.Contains(s) select s).Any();