Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial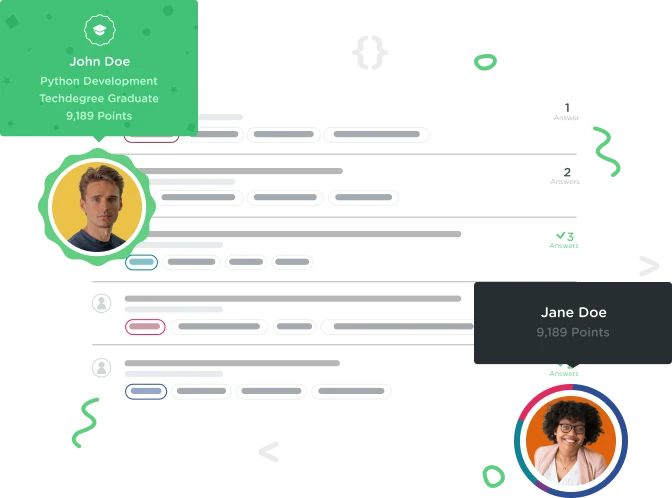

James Joseph
4,885 PointsList app [extra credit] open and save
Hey guys, I'm working on the extra credit project for the list app. I want to open the file after I've saved it. I got the save file patted down but I'm having trouble open it.
When I tried to open the file it had double brackets so I looked up online about .replace to remove brackets however I'm getting errors when opening it. Can someone advise what I'm doing wrong or what I can do to improve it?
#!/usr/bin/env python3
#printing intro
#version 0.1 - Basic app, typing DONE will close and print the app
#version 1.0 - Everything is now in functions, added elif statements
#version 1.1 - Adding save and open function to the list app
import os
from subprocess import call
print("Welcome to the list app!")
def show_help():
print('''To add an item into the list, type it in and hit return. For specific commands:
DONE - This will print the list and quit the application
SHOW - This will show the current list
HELP - This will show this explination of commands
SAVE - This will save the list into a text file of your choice
OPEN - This will open a previous saved list''')
def show_list():
print("Here is your list")
for items in fulllist:
print(items)
def add_to_list(listadd):
fulllist.append(listadd)
print("Adding {} to your list! You now have {} items in your list".format(listadd, len(fulllist)))
def savlist():
while True:
confch = input("Do you want to save the list? We will put it into the app directory (y/n) ")
if confch == "y":
filesave = open('pyapp.txt', 'w')
print("Saving file now!")
savl = str(fulllist)
for items in savl:
filesave.write(items)
filesave.close()
print("File saved!")
break
elif confch == "n":
continue
def openlst():
readls = input("Do you want to open your previous list? (y/n)")
if readls == "y":
print("Opening list now!")
filesave = open('pyapp.txt', 'r')
filesave = filesave.replace("[", "" )
filesave = filesave.replace("]", "" )
for items in filesave:
fulllist.read(items).split(",")
filesave.close()
print("List imported!")
else:
print("Not importing list, continue adding items!")
show_help()
#efining list
fulllist = []
#inputting items
while True:
listadd = input("> ")
#quitting the app
if listadd == "DONE":
break
#showing the list
elif listadd == "SHOW":
show_list()
continue
#help menu for the app
elif listadd == "HELP":
show_help()
continue
elif listadd == "SAVE":
savlist()
continue
elif listadd == "OPEN":
openlst()
continue
#adding items onto the list
add_to_list(listadd)
#printing the list
show_list()