Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial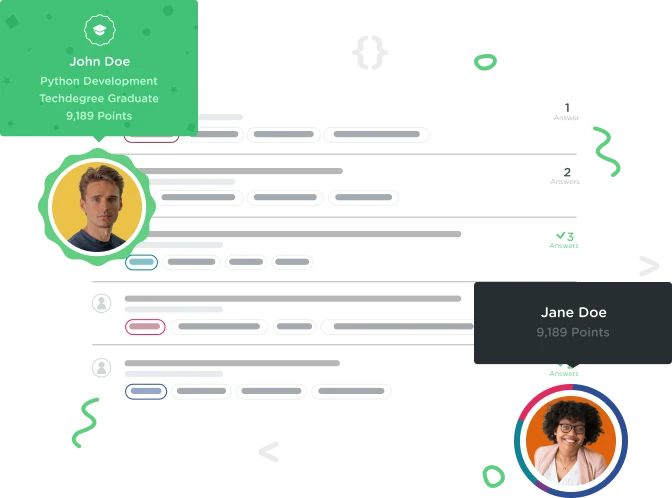
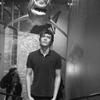
olegovich7
6,605 PointsList sorting
I can't really understand: if execute this in python shell:
>>>a = [5,4,3,2,1]
>>>b = a
>>>b.sort()
>>> b
[1, 2, 3, 4, 5]
>>> a
[1, 2, 3, 4, 5]
why a is also sorted?
3 Answers

Umesh Ravji
42,386 PointsHi olegovich7, that's because a
and b
are the same list. When you assign a
to b
, you aren't making a copy of the list, you are just making it point to the same place in memory. Performing an operation on either variable performs that operation on the single list in memory.
Instead you could make a copy, see the code below.
a = [5,4,3,2,1]
b = a[:]
b.sort()
print(a) # [5, 4, 3, 2, 1], sorting b has no effect on a

andren
28,558 PointsBecause this line:
b = a
Makes b
and a
contain the same list. Not just the same list content, but literally the same list.
In Python when you assign a list to a variable you in reality assign it a reference to a point in memory where the list is stored. When you set another variable equal to that list what actually gets set to the variable is not the contents of the list but the reference to the list. Meaning that both variables now point to the same location in memory.
This means that in reality while there are two variables pointing at the list there is only one actual list existing. So any modifications to that list, be it through variable a
or variable b
will end up changing that list for both of them.
This is a pretty standard behavior you will find in a lot of languages, certain data types will pass a memory reference when you use them and some will pass the value they store.
So far you have mostly worked with value-based types like string and int. It's worth mentioning that some of this is a bit simplified but it will hopefully get you to somewhat understand what is going on.
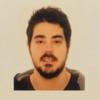
NARCIS MATABOSCH
1,540 PointsThank you very much for these posts because I didn't understand the use of [:] to copy a list =)