Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial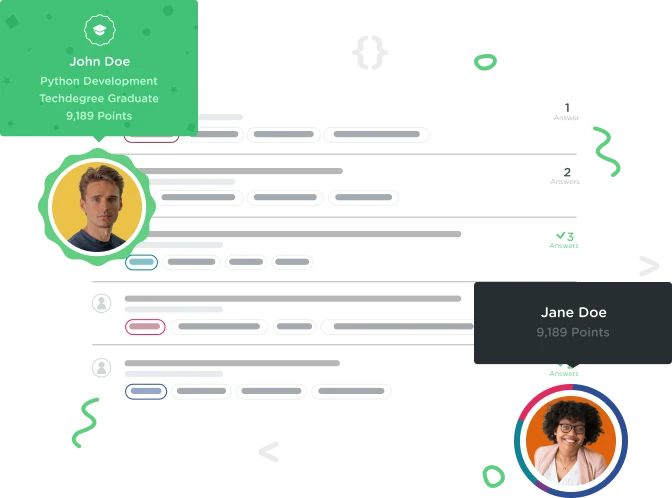

shawn rashirai
1,104 Pointslists code challenge
This is the code challenge OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end.
I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!
Oh, be sure to look for both uppercase and lowercase vowels!
This how I went about trying to solve the puzzle a,b,c,d,e='a','e','i','o','u' x= a,b,c,d,e def disemvowel(word): word=list(word) for a in word: word.remove(a) for b in word: word.remove(b) for c in word: word.remove(c) for d in word: word.remove(d) for e in word: word.remove(e) return word
This is the code challenge
OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end.
I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!
Oh, be sure to look for both uppercase and lowercase vowels!
This how I went about trying to solve the puzzle
a,b,c,d,e='a','e','i','o','u'
x= a,b,c,d,e
def disemvowel(word):
word=list(word)
for a in word:
word.remove(a)
for b in word:
word.remove(b)
for c in word:
word.remove(c)
for d in word:
word.remove(d)
for e in word:
word.remove(e)
return word
1 Answer
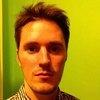
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsFirst of all, there's a much more efficient way of creating a list:
vowels = ['a', 'e', 'i', 'o', 'u']
As far as the challenge, when you loop through the word with this code:
for a in word:
It's going to loop through every character in the list, and and put that variable into the temporary variable a
. Not just the a's are going to show up, all the letters will. You should probably give it a different variable name for clarity. Maybe:
for letter in word:
Then you'll need some logic to check if that letter is a vowel before you remove it.
Adam Gataev
9,633 PointsAdam Gataev
9,633 PointsHello, I tried this but it isn't correct could you help me I would appreciat it.
def disemvowel(word): word = word.lower() vowels = ['a','e','i','o','u'] if list in word: word.remove(vowels) return word
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsYou're referring to a
list
variable, but it doesn't seem like you declared it. Also, I think you want to loop through the word, and check if the character is a vowel.