Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial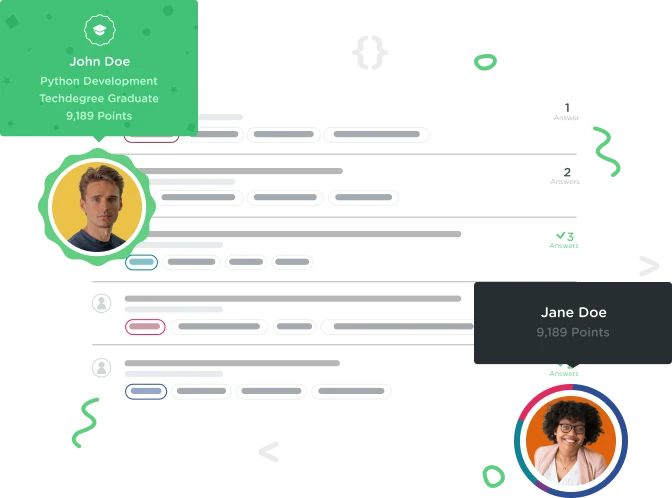
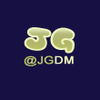
Jonathan Grieve
Treehouse Moderator 91,253 PointsLists Interfaces - Java
Again, this is based off the videos, documentation and notes that I made. I hope I'm not too far off because I think going back and writing these notes from the last view videos has been very useful. I don't quite get everything yet but that's a far cry from 2 weeks ago when this stuff in this course went completely over my head and I was bundling through.
Lists
The first interface in the collection is a List Interface. - http://docs.oracle.com/javase/8/docs/api/java/util/List.html
Lists are an ordered collection of items. In Java Lists, you can insert or replace items wherever you want to by index within a zero based index. You can check if something is in a list and sort it.
There are ArrayLists and LinkedLists.
ArrayList implements the list interface. You usually start with using ArrayLists.
To use Lists you need to implement something like List<String> this example is a "List of Strings"
List<String> fruit = new ArrayList<String>();
//using a method on an arrayList
fruit.isEmpty();
To use Lists you need to implement something like List<String> this example is a "List of Strings"
.add()
.remove()
.size()
.isEmpty()
.contains()
.add(1, "element to add here");
Collections.sort(fruit); //interface must be Comparable
e.g.
List<String> fruit = new ArrayList<String>();
implementation definition
To return an Object Array to a String Array()
fruit.toArray();
fruit.toArray(new String[0]);
asList() pass in a String array and return it as a List.
Using ArrayLists
import java.util.ArrayList;
import java.util.List; import the List Interface.
//return a list of strings that start with #
public List<String> getHashTags() {
//store a new list of strings using List.
List<String> results = new ArrayList<String>();
//loop through a set of words in a Treet getWords method
for(String word : getWords()) {
//dynamically add words to the list if they begin with a #
if(word.startsWith("#")) {
results.add(word);
}
}
return results;
}
//code for getting mentions
public List<String> getMentions() {
List<String> results = new ArrayList<String>();
for(String word : getWords()) {
//dynamically add words to the list if they begin with a @
if(word.startsWith("@")) {
results.add(word);
}
}
return results;
}
instead use methods like this
public List<String> getHashTags() {
return getWordsPrefixedWith("#");
}
public List<String> getMentions() {
return getWordsPrefixedWith("@");
}
public List<String> getWordsPrefixedWith(String prefix) {
List<String> results = new ArrayList<String>();
for(String word : getWords()) {
//dynamically add words to the list if they begin with a @
if(word.startsWith(prefix)) {
results.add(word);
}
}
return results;
}
Object References
When we add objects to a collection, arrays included, we're actually adding the Object reference.
A reference is used to point to a specific place in memory.
When object values are changed they're kept because they're references to points in memory.
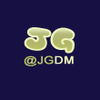
Jonathan Grieve
Treehouse Moderator 91,253 PointsThat's great to hear Nelson, thanks! :)
Nelson Fleig
25,764 PointsNelson Fleig
25,764 PointsThank you for the reference Jonathan. I am having trouble putting the pieces together and this has helped a lot!