Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial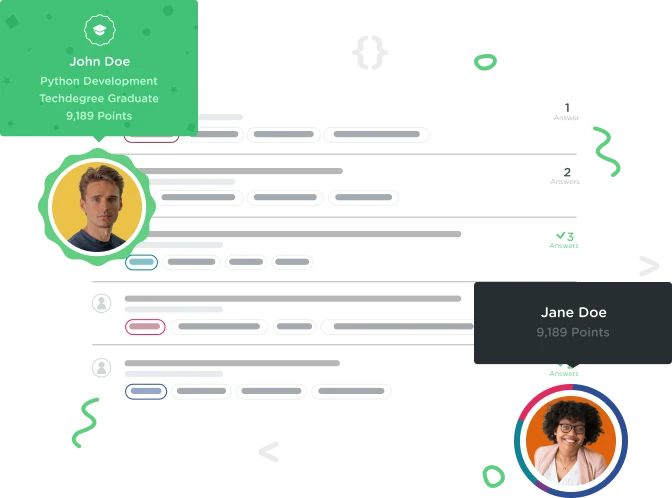

Craig Fender
7,605 PointsLiteral Boolean Value in Loops
I've seen this used a few times where a literal Boolean value is in the loop, like this...
while (true) { ... }
Prior to taking this course on JavaScript, I really haven't seen this used before in either Java, C#, or PHP. I'm wondering if this is a preferred method to using while and do...while loops because it's simpler and cleaner than using some Boolean variable that you can set to false in the loop block. Or maybe this is good to only use with JavaScript. Or maybe it's not a good practice to use at all and it's only been used for educational purposes right now. Can anyone clear this up for me? I'm always looking for ways to improve my code. Thanks.
1 Answer
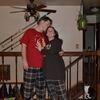
Julie Myers
7,627 Pointswhile(true){...} is usually used only as a learning tool on how to make your loop never stop running and eventually crash the browser.
In order to get a loop to run the conditional needs to be true. In order to get a loop to stop the conditional needs to be turned to false. Loop conditionals only accept a Boolean value, which can come in many forms. For example:
var count = 0;
while(count < 4) {
console.log(count);
count++;
}
/*
This while loop will continue to run (it's conditional is true) until the count
variable is 5. Once count=5 the loop's condition will turn to false and the
loop will stop running.
*/
/*
There are things called truthy and falsy values. It's easy to show the limited
number of falsy values. Look at this example:
*/
function logTruthiness (val) {
if (val) {
console.log("Truthy!");
} else {
console.log("Falsy.");
}
}
// Outputs: "Falsy."
logTruthiness(false);
// Outputs: "Falsy."
logTruthiness(null);
// Outputs: "Falsy."
logTruthiness(undefined);
// Outputs: "Falsy."
logTruthiness(NaN);
// Outputs: "Falsy."
logTruthiness(0);
// Outputs: "Falsy."
logTruthiness("");
// Outputs: "Truthy!"
logTruthiness(25);
You will use the for loop way more then the while loop. And you will commonly use the for..in loop for iterating through objects.