Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial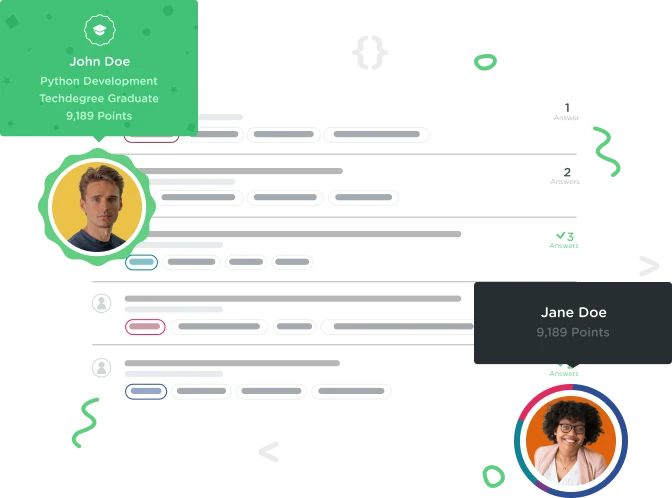

Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsLittle help, please?
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
boolean isHit = prompter.promptForGuess();
if (isHit) {
System.out.println("You got a hit!");
} else {
System.out.println("Whoops, you missed!");
}
}
}
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
public class Game {
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mAnswer += letter;
} else {
mMisses += letter;
}
}
}
I get an error from the console telling:
./Game.java:18: error: missing return statement
}
^
Please advise.
1 Answer
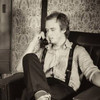
Jan Van Raemdonck
22,961 PointsThe error is telling you exactly what you need to know. you are missing a return statement. your function definition is
public boolean applyGuess(char letter)
which means you will be returning a boolean. However, you are not returning anything in the function. To solve this problem, you either have to change boolean to void, which means you don't want to return anything. Or you have to add
return isHit;
to the end of the applyGuess function, assuming you want to return the value of isHit.
Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsHariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsThanks a lot, Jan Van Raemdonck!