Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial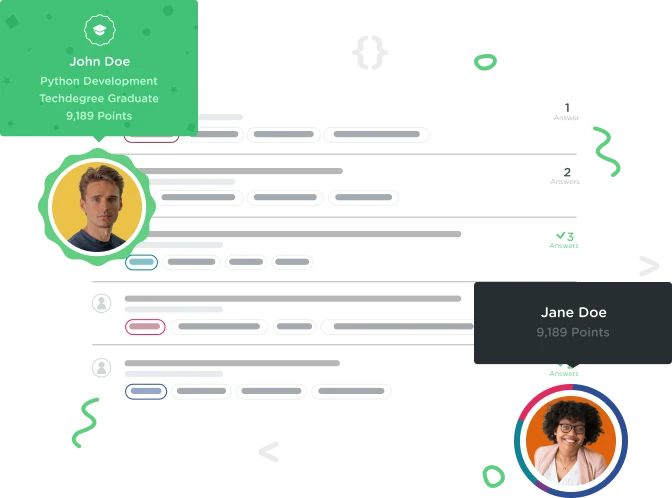

Alex Hedley
16,381 PointsLoad Artists for ComicBook
In program.cs there is a method
private static void ListComicBook(int comicBookId)
This loads the comicbook then loops the Artists:
ComicBook comicBook = Repository.GetComicBook(comicBookId);
foreach (ComicBookArtist artist in comicBook.Artists)
{
ConsoleHelper.OutputLine("{0} - {1}", artist.Artist.Name, artist.Role.Name);
}
If we wanted to add a Repository method to return a List of Artists can this be done in Linq?
public IEnumerable<Artist> GetArtistsForComicBook(int comicBookId)
We have
return context.ComicBooks
.Include(cb => cb.Artists)
.Where(cb => cb.Id == comicBookId)
.SingleOrDefault();
Can you make a Select to just return the cb.Artists.Artist List Since cb.Artists is a ComicBookArtist and I actually want a List of Artist
1 Answer
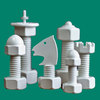
Steven Parker
229,771 PointsI believe you can do this with a one-liner.
You don't need LINQ just to get a list:
return Repository.GetComicBook(comicBookId).Artists.ToList();
Alex Hedley
16,381 PointsAlex Hedley
16,381 PointsI'm getting the following error at first go, but I'll look into it
Steven Parker
229,771 PointsSteven Parker
229,771 PointsYou could cast the result, or just define the method to return a list: