Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial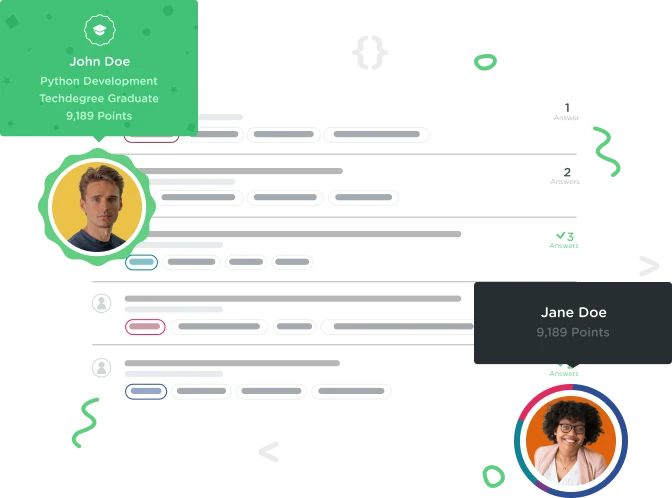

German Espitia
4,578 PointsLoad images before view is loaded
So I have two views, view A and view B. View B is a UITableViewController
where images of friends of the current user will be loaded (think the feed for instagram).
I want to call a method in view A in it's viewDidLoad
that starts loading the images that will appear in view B.
What I was trying to do is get an instance of B and call it from A to start loading the images and saving those images in an array in class B.
ClassB *classB = [ClassB alloc] init];
[classB loadInitialImages];
When the user gets to class B tho, that array is empty. What I think is wrong is that I'm getting an instance of B so when a user get to B, they are loading a different instance.
2 Answers

Derek Stepan
1,954 PointsOh, I see what you mean. I saw UITableViewController and I made an assumption.
This may be helpful. http://stackoverflow.com/questions/9955344/how-to-send-uitabbarcontroller-one-tab-view-textfield-value-in-to-another-tab-vi
It looks like maybe the instance is created by the UITabBar so you need to get a handle on the view controller from there. Let me know if you figure it out!

Derek Stepan
1,954 PointsIn your didSelectRowAtIndexPath method, you're probably creating a new instance of that view controller and then pushing the view controller onto the stack (that's how I normally do it anyway). If this is the case, then your assumption about a different instance is correct. What might work for you is to store the images in an NSArray in ClassA and then before pushing the view controller for ClassB onto the stack, set the array.
// In ClassA viewDidLoad
ClassB *classB = [[ClassB alloc] init];
[classB loadInitialImages];
self.imageArray = classB.imageArray;
// In didSelectRowAtIndexPath
ClassB *classB = [[ClassB alloc] init];
classB.imageArray = self.imageArray;
// push view controller
This can be cleaned up a lot, but it's the best I could do since I don't have Xcode in front of me.
Edit: It may be more beneficial for you to make the loadInitialImages method common and return an NSArray so it can be accessed by both ClassA and ClassB. That way you don't have to initially create an instance of ClassB in viewDidLoad, you can create an instance of a common ViewController which would have a lot of other common methods in there as well

German Espitia
4,578 PointsPushing on to the stack means via a segue right? My case is different. I have a tabBarController which has 2 tabs. One for ClassA and another for ClassB meaning I cant access it by doing prepareForSegue
.
Also, I am not using didSelectRowAtIndexPath
at any point.
Sorry if I wrote the question wrong, I'm simply trying to access another class and loading certain images on to it.

Derek Stepan
1,954 PointsMy below answer was supposed to be in response to this comment. I'm newbie to this forum.
German Espitia
4,578 PointsGerman Espitia
4,578 PointsThank you! That link lead me in the right way. I had to first access the tabBarController's viewControllers and then acess the navigationController's views.