Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial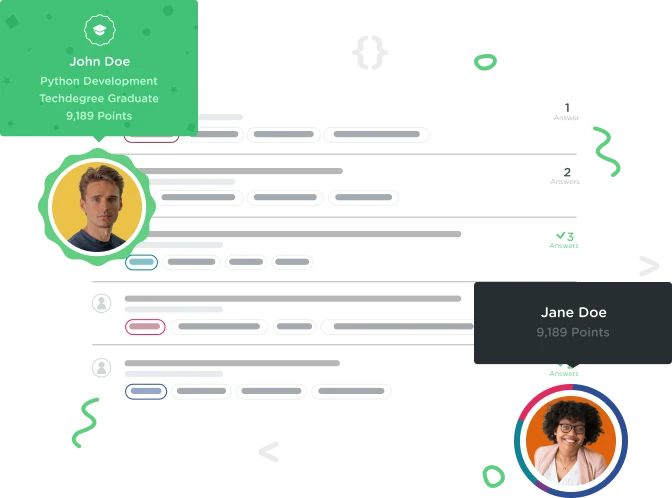

Sean Hayes
Courses Plus Student 8,106 PointsLoadException
So I have watched this video many times, and run countless Google searches but I cannot figure out what the problem is.
Main.java
package sample;
import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.stage.Stage;
public class Main extends Application{ @Override public void start(Stage primaryStage) throws Exception{ Parent root = FXMLLoader.load(getClass().getResource("sample.fxml")); primaryStage.setTitle("Sup"); primaryStage.setScene(new Scene(root,300,275)); primaryStage.show(); }
public static void main(String[] args){
launch(args);
}
}
sample.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.geometry.Insets?> <?import javafx.scene.layout.GridPane?>
<?import javafx.scene.control.Button?> <?import javafx.scene.control.Label?> <?import javafx.scene.control.Text?> <?import javafx.scene.control.TextField?>
<GridPane fx:controller="sample.Controller" xmlns:fx="http://javafx.com/fxml" alignment="center" hgap="10" vgap="10">
<Text text="Sup"
GridPane.rowIndex="0"
GridPane.columnSpan="2"
GridPane.halignment="CENTER"></Text>
<!--<Label text="First Name:"
GridPane.rowIndex="1"
GridPane.columnIndex="0"></Label>
<TextField
GridPane.columnIndex="1"
GridPane.rowIndex="1"></TextField>
<Button text="Say Sup!"
onAction="#handleSaySup"
GridPane.rowIndex="2"
GridPane.columnIndex="1"
GridPane.halignment="RIGHT"></Button> -->
</GridPane>
And the Error:
Exception in Application start method java.lang.reflect.InvocationTargetException at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.sun.javafx.application.LauncherImpl.launchApplicationWithArgs(LauncherImpl.java:389) at com.sun.javafx.application.LauncherImpl.launchApplication(LauncherImpl.java:328) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at sun.launcher.LauncherHelper$FXHelper.main(Unknown Source) Caused by: java.lang.RuntimeException: Exception in Application start method at com.sun.javafx.application.LauncherImpl.launchApplication1(LauncherImpl.java:917) at com.sun.javafx.application.LauncherImpl.lambda$launchApplication$155(LauncherImpl.java:182) at java.lang.Thread.run(Unknown Source) Caused by: javafx.fxml.LoadException: /C:/Users/1126386/workspace/FirstJavaFX/bin/sample/sample.fxml
at javafx.fxml.FXMLLoader.constructLoadException(FXMLLoader.java:2601)
at javafx.fxml.FXMLLoader.importClass(FXMLLoader.java:2848)
at javafx.fxml.FXMLLoader.processImport(FXMLLoader.java:2692)
at javafx.fxml.FXMLLoader.processProcessingInstruction(FXMLLoader.java:2661)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2517)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2441)
at javafx.fxml.FXMLLoader.load(FXMLLoader.java:2409)
at sample.Main.start(Main.java:13)
at com.sun.javafx.application.LauncherImpl.lambda$launchApplication1$162(LauncherImpl.java:863)
at com.sun.javafx.application.PlatformImpl.lambda$runAndWait$175(PlatformImpl.java:326)
at com.sun.javafx.application.PlatformImpl.lambda$null$173(PlatformImpl.java:295)
at java.security.AccessController.doPrivileged(Native Method)
at com.sun.javafx.application.PlatformImpl.lambda$runLater$174(PlatformImpl.java:294)
at com.sun.glass.ui.InvokeLaterDispatcher$Future.run(InvokeLaterDispatcher.java:95)
at com.sun.glass.ui.win.WinApplication._runLoop(Native Method)
at com.sun.glass.ui.win.WinApplication.lambda$null$148(WinApplication.java:191)
... 1 more
Caused by: java.lang.ClassNotFoundException: javafx.scene.control.Text at java.net.URLClassLoader.findClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source) at java.lang.ClassLoader.loadClass(Unknown Source) at javafx.fxml.FXMLLoader.loadTypeForPackage(FXMLLoader.java:2916) at javafx.fxml.FXMLLoader.loadType(FXMLLoader.java:2905) at javafx.fxml.FXMLLoader.importClass(FXMLLoader.java:2846) ... 15 more Exception running application sample.Main
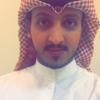
Fahad Mutair
10,359 Pointsso hard to read your code would be better to use Markdown cheatsheet
11 Answers

Sean Hayes
Courses Plus Student 8,106 PointsMain.java
package sample;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application{
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("../sample/sample.fxml"));
primaryStage.setTitle("Sup");
primaryStage.setScene(new Scene(root,300,275));
primaryStage.show();
}
public static void main(String[] args){
launch(args);
}
}
sample.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.layout.GridPane?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.Text?>
<?import javafx.scene.control.TextField?>
<GridPane fx:controller="sample.Controller"
xmlns:fx="http://javafx.com/fxml" alignment="center" hgap="10" vgap="10">
<Text text="Sup"
GridPane.rowIndex="0"
GridPane.columnSpan="2"
GridPane.halignment="CENTER"></Text>
<!--<Label text="First Name:"
GridPane.rowIndex="1"
GridPane.columnIndex="0"></Label>
<TextField
GridPane.columnIndex="1"
GridPane.rowIndex="1"></TextField>
<Button text="Say Sup!"
onAction="#handleSaySup"
GridPane.rowIndex="2"
GridPane.columnIndex="1"
GridPane.halignment="RIGHT"></Button> -->
</GridPane>
Error Message
Exception in Application start method
java.lang.reflect.InvocationTargetException
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)
at com.sun.javafx.application.LauncherImpl.launchApplicationWithArgs(LauncherImpl.java:389)
at com.sun.javafx.application.LauncherImpl.launchApplication(LauncherImpl.java:328)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source)
at java.lang.reflect.Method.invoke(Unknown Source)
at sun.launcher.LauncherHelper$FXHelper.main(Unknown Source)
Caused by: java.lang.RuntimeException: Exception in Application start method
at com.sun.javafx.application.LauncherImpl.launchApplication1(LauncherImpl.java:917)
at com.sun.javafx.application.LauncherImpl.lambda$launchApplication$155(LauncherImpl.java:182)
at java.lang.Thread.run(Unknown Source)
Caused by: javafx.fxml.LoadException:
/C:/Users/1126386/workspace/FirstJavaFX/bin/sample/sample.fxml
at javafx.fxml.FXMLLoader.constructLoadException(FXMLLoader.java:2601)
at javafx.fxml.FXMLLoader.importClass(FXMLLoader.java:2848)
at javafx.fxml.FXMLLoader.processImport(FXMLLoader.java:2692)
at javafx.fxml.FXMLLoader.processProcessingInstruction(FXMLLoader.java:2661)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2517)
at javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:2441)
at javafx.fxml.FXMLLoader.load(FXMLLoader.java:2409)
at sample.Main.start(Main.java:13)
at com.sun.javafx.application.LauncherImpl.lambda$launchApplication1$162(LauncherImpl.java:863)
at com.sun.javafx.application.PlatformImpl.lambda$runAndWait$175(PlatformImpl.java:326)
at com.sun.javafx.application.PlatformImpl.lambda$null$173(PlatformImpl.java:295)
at java.security.AccessController.doPrivileged(Native Method)
at com.sun.javafx.application.PlatformImpl.lambda$runLater$174(PlatformImpl.java:294)
at com.sun.glass.ui.InvokeLaterDispatcher$Future.run(InvokeLaterDispatcher.java:95)
at com.sun.glass.ui.win.WinApplication._runLoop(Native Method)
at com.sun.glass.ui.win.WinApplication.lambda$null$148(WinApplication.java:191)
... 1 more
Caused by: java.lang.ClassNotFoundException: javafx.scene.control.Text
at java.net.URLClassLoader.findClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at javafx.fxml.FXMLLoader.loadTypeForPackage(FXMLLoader.java:2916)
at javafx.fxml.FXMLLoader.loadType(FXMLLoader.java:2905)
at javafx.fxml.FXMLLoader.importClass(FXMLLoader.java:2846)
... 15 more
Exception running application sample.Main

Sean Hayes
Courses Plus Student 8,106 Pointssrc ->sample ->->Main.java ->->sample.fxml
I've tried the code getResource("../sample/sample.fxml")); and getResource("sample.fxml")); both with no luck

Sean Hayes
Courses Plus Student 8,106 PointsI moved the folder, no luck
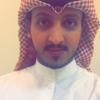
Fahad Mutair
10,359 PointsDid you mark it as resource directory

Sean Hayes
Courses Plus Student 8,106 PointsI'm not seeing how to mark it as a resource folder. How do you mark it?
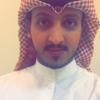
Fahad Mutair
10,359 Pointsi just review your code and compare it to the code in the video Why did you comment this line?
<!--<Label text="First Name:"
GridPane.rowIndex="1"
GridPane.columnIndex="0"></Label>
<TextField
GridPane.columnIndex="1"
GridPane.rowIndex="1"></TextField>
<Button text="Say Sup!"
onAction="#handleSaySup"
GridPane.rowIndex="2"
GridPane.columnIndex="1"
GridPane.halignment="RIGHT"></Button> -->
remove those <!-- and --> and keep your sample.fxml back dont forget to set this line also as
getResource("sample.fxml"));
so copy and paste this in ur
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.layout.GridPane?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.Text?>
<?import javafx.scene.control.TextField?>
<GridPane fx:controller="sample.Controller"
xmlns:fx="http://javafx.com/fxml" alignment="center" hgap="10" vgap="10">
<Text text="Sup"
GridPane.rowIndex="0"
GridPane.columnSpan="2"
GridPane.halignment="CENTER"></Text>
<Label text="First Name:"
GridPane.rowIndex="1"
GridPane.columnIndex="0"></Label>
<TextField
GridPane.columnIndex="1"
GridPane.rowIndex="1"></TextField>
<Button text="Say Sup!"
onAction="#handleSaySup"
GridPane.rowIndex="2"
GridPane.columnIndex="1"
GridPane.halignment="RIGHT"></Button>
</GridPane>

Sean Hayes
Courses Plus Student 8,106 PointsSo I created a resources folder, moved the sample.fxml file into that folder.
In the parentheses for getResource, I have tried the following:
- "resources/sample.fxml"
- "/resources/sample.fxml"
- "../resources/sample.fxml"
- "/sample.fxml"
- "sample.fxml"
None of these work. I am working with Eclipse. Folder structure:
FirstJavaFX
- src
- sample
- Controller.java
- Main.java
- resources
- sample.fxml
- sample
No other major changes to the code I posted earlier
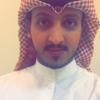
Fahad Mutair
10,359 PointsI recommend you to work on Intellij Better than Eclipse, i changed the commented lines only

Sean Hayes
Courses Plus Student 8,106 PointsYeah, I'm thinking that's the path I'm going to have to take. Thanks for your help
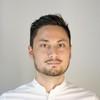
lio1986
19,649 PointsActually I got the same error with Netbeans, switched to IntelliJ and I still get the same error.

Jose Galvez
1,246 PointsDon't know if this will help, but my problem was not including
package sample;
on my Controller.java. It needs to be on both main.java and controller.java but not in sample.fxml
again, hopefully this solved your issue, I was about to lose my mind!
Edit: I encountered more errors, I was able to clear them up by declaring a "Resources" folder as an actual resource by going into File -> Project Structure -> Modules -> Resources folder, right click and select "Resources"

reggaeshark
8,542 PointsThe problem is it's trying to load the file fxml file from compiled files at runtime. eg. Intellij generates target directory with compiled classes and the file is often copied to an incorrect location.
https://mkyong.com/java/java-read-a-file-from-resources-folder/
workaround. I pul my non-source-files into resources in IntellijProject.
1) load the URL with utility method
URL fxmlURL = FileResourcesUtils.getUrlOfFileFromeResource("sample.fxml");
2) pass it to the FXMLLoader.load(URL)
Parent root = FXMLLoader.load(fxmlURL);
code for getting the URL
public class FileResourcesUtils { public static URL getUrlOfFileFromeResource(String fileName) throws URISyntaxException {
ClassLoader classLoader = FileResourcesUtils.class.getClassLoader();
URL resource = classLoader.getResource(fileName);
if(resource == null) {
throw new IllegalArgumentException("file not found!" + fileName);
} else {
return resource;
}
}
}
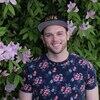
Dominick DeChristofaro
3,219 PointsIf you get a NullPointerException while following this video, it means that intelliJ is having trouble finding your file.
In the video example, it shows the sample.fxml
file in the root of the folder structure. But intelliJ is was looking in the resources.<folder_structure>.sample.fxml in my case.
Try moving your sample.fxml file and re-running the program to see if it resolves the NullPointerExpection.
Sean Hayes
Courses Plus Student 8,106 PointsSean Hayes
Courses Plus Student 8,106 PointsAnd I have no clue why the format came out so weird...