Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial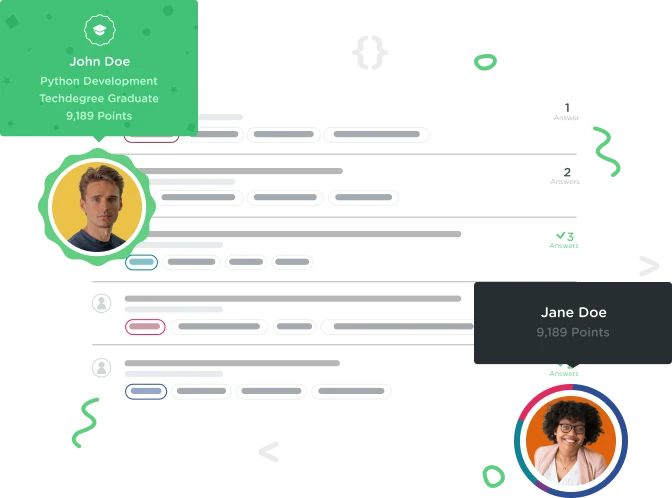

Chaz Hall
1,970 Pointsload(MAX_PEZ); Please Explain Like I Am 5 years old
public void load (){ load(MAX_PEZ); } public void load(int pezAmount){ mPezCount+=pezAmount; }
This may be long winded, so I apologize, but i'm struggling with this concept. I understand that we've declared load as a method variable. I do not understand what load(MAX_PEZ) 's function is thought. What do the parenthysis signify? With that answered, why is load in front of it?
With the next method, load then has in parenthesis int pezAmount, which is an integer variable and says that it becomes mPezCount=mPezCount+pezAmount. Is this method somehow referencing MAX_PEZ, which is set to 12 in these example?
Does this mean that pezAmount is set to 12 because of the first method stating load(MAX_PEZ)? I am terrible with the terms and more or less want non java logic explanations, it's just how I have been understanding it so far. Thanks!
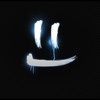
Grigorij Schleifer
10,365 PointsIf you are in a different class you will need to create an instanse of the PezDispenser class and call it f.e. "dispenser" (see Shadds answer) .
PezDispenser dispenser = new PezDispenser();
// creating a new PezDispenser object called dispenser
dispenser.load();
// would call the load without parameters
// and fiill the dispenser to the top ( the lazy way)
or
dispenser.load(MAX_PEZ);
// would call the load with parameters
// and do the same as the method above
// but you will nee to type MAX_PEZ into the parenthesis
If you are in the PezDispenser class you don´t need to create a new object and you can call
load();
// would call the load without parameters
load(12);
or
load(MAX_PEZ);
// would call the load with parameters
Don´t forget that programmers are the laziest peaple on earth !!!
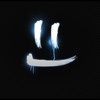
Grigorij Schleifer
10,365 PointsHey boys,
lets go hunting :)))))
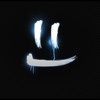
Grigorij Schleifer
10,365 PointsHey :)
dispenser.load();
// will fill the PezDispenser to the top
dispenser.load(pezAmount);
// will fill the PezDispenser in dependance of the value that is stored inside pezAmount
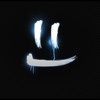
Grigorij Schleifer
10,365 PointsYeeeeee !
Time for a break
5 Answers
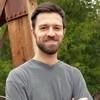
Shadd Anderson
Treehouse Project ReviewerYou're close! Basically, to explain it a little further, the second load method he created takes a parameter (the "int pezAmount" in the parentheses). What that means is that whenever someone calls the .load method, they now have two options. They can either do load(some number), or load() - with nothing in the parentheses. So for the first one, for example, if someone does:
dispenser.load(3);
the dispenser will then add 3 PEZ. If someone does this:
dispenser.load();
//note, nothing in the parentheses
then the dispenser will be filled to the top. This is because when he changed the first method to load(MAX_PEZ), he basically created a method that does load(12) - because that's what the value of MAX_PEZ is.
All that make sense?
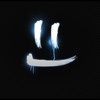
Grigorij Schleifer
10,365 PointsCool answer !
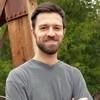
Shadd Anderson
Treehouse Project ReviewerHa! Good to know at least my answer can be backed up :D
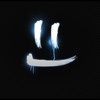
Grigorij Schleifer
10,365 PointsHey Shadd,
fully backed up !!! 12 points :)))

Chaz Hall
1,970 Pointsit is helping for sure. I'm struggling with the += operator because I don't understand what it is actually doing. Is the load method with parameters going to set 12 to int pezAmount?
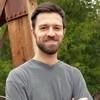
Shadd Anderson
Treehouse Project ReviewerThe only thing the "+=" does is ADD whatever the number is, and then set the new number EQUAL to what that result is. So for instance, if the dispenser has 2 PEZ in it, and you call dispenser.load(3), it will ADD 3, and then set the amount to 2+3, or 5.

Chaz Hall
1,970 PointsUsing the method of PezDispenser (which tells us that mPezcount is 0) Would you refer to the load method as PezDispenser.load() and the other one as PezDispenser.load(int pezAmount)?
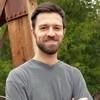
Shadd Anderson
Treehouse Project ReviewerWhat may be confusing you is the fact that there's two methods, basically doing the same thing. The only difference is whether or not you want to do a specific amount of PEZ, or if you want it to just fill to the top, regardless of where it's currently at. So the one, load(), without anything in the parentheses, is the "lazy" way, to just fill it to the top. load(int pezAmount), is going to be the one that's more specific. You can call either of these, it just depends on whether you want to just throw a couple in there or fill it all the way up.

Chaz Hall
1,970 PointsI think of it as a gun and an ammo clip. If the clip holds 12 bullet once those are gone it automatically reloads. But sometimes you want to load the clip even if it's only lost 1 bullet. In a video game the user can choose to refill it before the ammunition is gone, but if they forget (like everyone does) it will automatically reload.
Using this example, i'm understanding that the load() and the load(int pezAmount) are doing a similar thing. mPezCount is going mPezCount=mPezCount+pezAmount. Well if pezAmount is only in the load(int pezAmount) then won't it always be 0=0+0?
I'm sorry i'm having trouble understanding this. I'm so close to understanding it though and I appreciate the help.
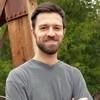
Shadd Anderson
Treehouse Project ReviewerYou're close! The difference, is this is talking about the CLIP of the gun, not the gun itself. The load() method (no specific integer) just fills it to the top, whether it's full or not, similar to someone reloading their gun before it's actually empty. You can think of the load(integer) method as manually putting in, say, 3 bullets to the clip.
Another way to visualize this:
You have a revolver. With revolvers, you have to put bullets manually into the gun. If you did revolver.load(), what this is saying, is that you fill up the gun with as many as can fit. It's unspecified exactly how many that is. However, if you did revolver.load(3), you only put 3 bullets in there, because you specified that you only put 3 in there.

Chaz Hall
1,970 PointsIs this how it is referenced from other things???
such as: dispenser.load() and another one as dispenser.load(pezAmount) ???
My confusion is from how it is referenced. If the above is correct, in that you reference the parameters also, then I finally get it! If not, time for a break.

Chaz Hall
1,970 PointsI think I finally understand it! Even though it took 6 hours! lol
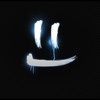
Grigorij Schleifer
10,365 PointsHi Chaz,
don´t give up !!!!!!!!!
Lets go over the code.
You have two different load() methods. The first one has no argument inside the parenthesis. That meas that it takes no parameters (nothing inside () ). The second one accepts an integer (here MAX_PEZ = 12). So it takes a number as argument/parameter, This number is the amount of Pez you want to load into the PezDispenser.
Important to know that we don´t declare a load method variable inside the first load method but we call the load method that takes the maximum number of Pez (=MAX_PEZ) inside the load() method that takes no parameters. I know it sounds strange.
// so if you call the first load() method
// the compiler takes the second load method
// that accepts the number of Pez here maximum pez number (=12)
// and adds it to the variable called "mPezCount"
public void load (){
load(MAX_PEZ);
}
public void load(int pezAmount){
mPezCount+=pezAmount;
}
For explanation purpose you can see it this way:
public void load (){
load(12);
}
public void load(int MAX_PEZ){
mPezCount+= MAX_PEZ;
}
Makes sense?
Grigorij

Chaz Hall
1,970 PointsThis is ALMOST making sense.
The load(int pezAmount), is the first method and addresses the MAX_PEZ issue and then the second load method says, "Okay, brother, what is the max pez we can hold? Okay, well i'm going to turn that value into an integer and use that number for my new variable pezAmount". Do I understand this right?
I don't understand why mPezCount says" mPezcount+=pezAmount ". Doesn't it automatically set it to 12 since it's the max and is what the first method load() is equal to? Since MAX_PEZ can't be changed and is set to 12 in this case, why even write it?
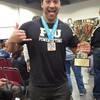
Gabriel Llanes
2,894 Pointsi understand how this works BUT why can't we just write this?:
public void load(){ mPezCount = MAX_PEZ; }
public void load(int pezAmount){ mPezCount = pezAmount; }
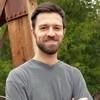
Shadd Anderson
Treehouse Project ReviewerYou absolutely can! The point of this was simply showing the fact that you can create multiple methods with the same name, just with different parameters, then you can use them within each other.
(Except the second method should be "mPezCount += pezAmount")

Steve Gold
1,402 Pointsokay so I was struggling to get it like you but I think I understand it now. Please correct me if I'm wrong. First, this is how I understand the second load method. We set that method to load however much we put into the parameters. So whatever number we put in the parenthesis that's how much it will load. So if we write "load(4); its gonna load 4. The way it knows how to do that is because of "mPezcount += pezAmount". Meaning it takes the whatever number is in "mPezCount" and adds whatever number is in "pezAmount" and the it makes "mPezCount" equal to that number. This is whats going on in the second "load" method. In the first load method, we set it that whenever we access it will in turn access the second method and it will automatically put "MAX_PEZ" (which is 12) in the parenthesis. This will make it that it will always load 12. So basically whenever we access the first load method we don't put anything in the parenthesis because it automatically has 12 in it. The first load method will be used for whenever the Pez thing is totally empty and the second load method will be used when you just want to load a certain amount. I hope I got this right:)
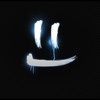
Grigorij Schleifer
10,365 PointsHey Tzvi,
you totally right !

Chaz Hall
1,970 PointsThank you all for the help. My summary of understanding.
Methods can have the same name as long as there is an argument. You reference each method independently by ensuring you type the method exactly as you have written it.
ammo() ammo(sniperRifle) ammo(shotgun) .etc
You all have been a great help on understanding this, thank you.
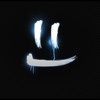
Grigorij Schleifer
10,365 PointsHey Chaz,
keep on going
See you in the forum
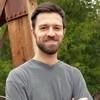
Shadd Anderson
Treehouse Project ReviewerProud of you
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey Chaz,
the mPezCount is also a number. It represents how many pez are inside the PezDispenser. If the PezDispenser is empty (mPezCount = 0) you can call
dispenser.load();
like Shadd has suggested. This method has the load(here comes the maximum number 12) method and sets the mPezCount to MAX_PEZ.