Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial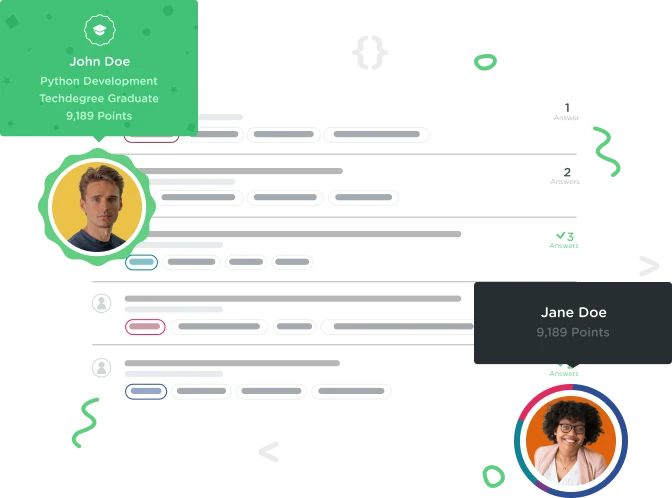

Dominic Bishop
Full Stack JavaScript Techdegree Graduate 15,302 PointsLocal storage: SOS please help me.
I've tried to implement the code from the local storage workshop into this code. But I cant seem to get it to work. I would of thought that you would need to target the UL after the code run and then call the functions. However it appears like always I am wrong. I have reset the code so it is similar to the one shown in the workshop.
const form = document.getElementById("registrar");
const input = form.querySelector("input");
const mainDiv = document.querySelector(".main");
const ul = document.getElementById("invitedList");
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckBox = document.createElement('input');
filterLabel.textContent = "Hide those who havent responded";
filterCheckBox.type = 'checkbox';
div.appendChild(filterLabel);
div.appendChild(filterCheckBox);
mainDiv.insertBefore(div, ul);
filterCheckBox.addEventListener("change", (e) => {
const isChecked = e.target.checked;
const lis = ul.children;
if (isChecked) {
for (let i = 0; i < lis.length; i += 1) {
let li = lis[i]
if (li.className === 'responded') {
li.style.display = '';
} else {
li.style.display = 'none';
}
}
} else {
for (let i = 0; i < lis.length; i += 1) {
let li = lis[i]
li.style.display = '';
}
}
});
/*
supportLocalStorage = () => {
try {
return "localStorage" in window && window["localStorage"] !== null;
}catch(e) {
return false;
}
}
getStoredListItems = () =>{
const storedItems = localStorage.getItem("storedItems");
if(storedItems) {
return JSON.parse(storedItems);
}
else {
return [];
}
}
saveStoredListItems = (str) => {
const storedItems = getStoredListItems();
storedItems.push(str);
localStorage.setItem("storedItems",JSON.stringify(storedItems));
}
removeListItem = (text) => {
localStorage.removeItem("storedItems",text);
*/
createLi = (text) => {
createElement = (elementName, property, value) => {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
appendElement = (elementName, property, value) => {
const element = createElement(elementName, property, value);
li.appendChild(element);
return element;
}
const li = document.createElement("li");
appendElement("span", "textContent", text);
appendElement("label", "textContent", "Confirmed")
.appendChild(createElement("input", "type", "checkbox"));
appendElement("button", "textContent", "edit");
appendElement("button", "textContent", "remove");
return li;
}
}
form.addEventListener("submit", (event) => {
event.preventDefault();
const text = input.value;
input.value = "";
const li = createLi(text);
ul.appendChild(li);
});
ul.addEventListener("change", () => {
const checkbox = event.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = "responded";
} else {
listItem.className = "";
}
});
ul.addEventListener("click", (e) => {
if (e.target.tagName === 'BUTTON') {
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
const action = button.textContent;
const nameActions = {
remove: () => {
ul.removeChild(li);
},
edit: () => {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'Save';
},
Save: () => {
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
}
};
nameActions[action]();
}
});
1 Answer

Joseph Bertino
Full Stack JavaScript Techdegree Student 14,652 PointsDominic,
The code as you provided above results in errors when running the webpage. There is an extra closing curly brace in the middle of the code, and you would benefit from improved indentation of your code.
Attached below is the local storage function I implemented in my code, and it works. Note that it's for a Form where each guest has a 3-way drop-down menu for the RSVP, rather than the "Confirm/Confirmed" checkbox. A good challenge for you would be to modify this code to be compatible with your form, where the guests have a checkbox.
/////// BEGIN LOCAL STORAGE FUNCTIONALITY
function supportsLocalStorage() {
try {
return 'localStorage' in window && window['localStorage'] !== null;
} catch (e) {
return false;
}
}
if (supportsLocalStorage()) {
var guestList = localStorage.getItem('guestList');
function getLocalGuestList() {
if (guestList) {
// return array of guests
return JSON.parse(guestList);
} else {
return [];
}
}
function addGuestFromLocal(guestArr) {
var newLI = document.createElement('li');
newLI.innerHTML = guestArr[0];
let selectedOpt = newLI.querySelector(`option[value="${guestArr[1]}"]`);
selectedOpt.selected = true;
let freetext = newLI.querySelector('input[type="text"]')
freetext.value = guestArr[2];
ul.appendChild(newLI);
}
// Initialize the List of Guests on window load.
window.onload = function() {
// Retrieve the list of guests from local storage
// and populate the
const loadGuestList = getLocalGuestList();
loadGuestList.forEach((guest) => {
addGuestFromLocal(guest);
});
}
window.addEventListener('beforeunload', (e) => {
// Save all guests from the list to local storage
// Eliminates the need to update guest list every time there is a change
const saveGuestList = []
const guests = ul.children; // Element.children returns an HTMLCollection... make it an array
console.log('money');
[...guests].forEach((guest) => {
/// Create the array to hold the guest's information
var guestArray = [guest.innerHTML];
/// Get the selected RSVP option for the guest
const selectedOpt = guest.querySelector('select').selectedOptions[0];
guestArray.push(selectedOpt.value);
// Get the guest's freetext from <input>
const freeText = guest.querySelector(`input[type="text"]`)
guestArray.push(freeText.value);
saveGuestList.push(guestArray);
});
localStorage.setItem('guestList', JSON.stringify(saveGuestList));
});
}
/////// END LOCAL STORAGE FUNCTIONALITY