Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial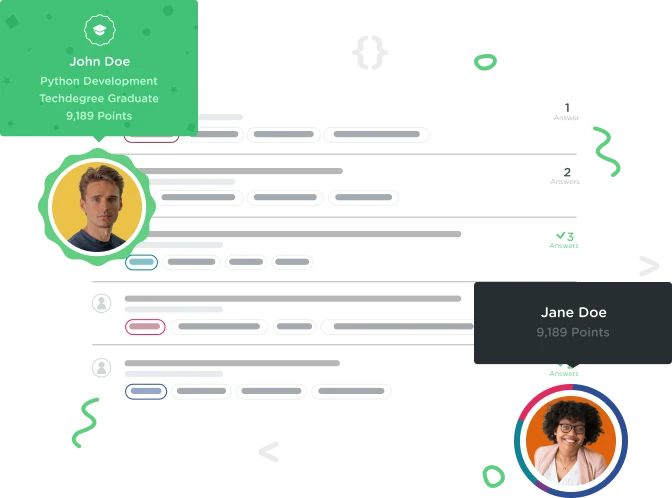

layeesolo
7,316 PointsLog an even number from 2 to 24 using loop.
The code below logs all of the even numbers from 2 to 24 to the JavaScript console. However, there's a lot of redundant code here. Re-write this using a loop. I can't get the number 12 on the console log. What am I doing wrong with this challenge?
for (var i = 2; i < 15; i++){
console.log(i);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
15 Answers
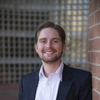
Stephen Van Delinder
21,457 PointsYou need to create a for loop that initializes the variable to 2, console.log the variable to the screen, and add two to the variable on each iteration.
Said another way: starting at two, as long as i is less than 25, add two and log the new variable to the screen.
for ( i=2 ; i<25 ; i+=2 ) {
console.log(i);
}
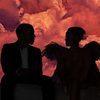
Basto Biih
5,334 PointsWhy don't you put in the variable everytime like they do in the videos??

Rainer Floeter
1,141 PointsI did this exact code and it didn't work.

Shafique Mohammed
Front End Web Development Techdegree Student 13,625 Pointsfor (i = 2; i <=24; i +=2) { console.log(i); }
This code worked fine for me

Cary Oldenkott
8,178 PointsWouldn't
for ( i = 2; i <= 24; i += 2;) { console.log(i); }
work just as well instead of i < 25?

Charlten Oldenkott
5,993 Pointsheeeeeellllllllllo sister!
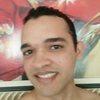
Rafael silva
23,877 Pointsno it's cause you need to add the number 25 like this:
for(i = 2 ; i <= 25 ; i += 2){ console.log(i); }
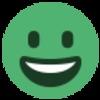
Jack Cummins
17,417 PointsAre you actually sisters?

Chris Drew
5,919 Pointsfor (var i = 2; i < 26; i += 2) {
console.log(i)
}
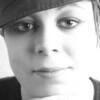
Leanne Millar
7,726 PointsThis works perfectly. Thanks, Chris Drew. Think this particular challenge was a little buggy.

Henry Chan
4,416 Pointsfor (i=2; i < 25; i++,i++) { console.log(i); }

Dipika Purohit
Courses Plus Student 20,955 Pointsfor ( i=2 ; i<25 ; i+=2 ) { console.log(i); }
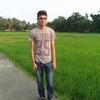
Joseph Graham
Courses Plus Student 845 Pointsvery easy
for ( var i = 2 ; i <= 24 ; i++ ) { if ( i % 2 === 0 ) { console.log (i);
}
}
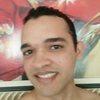
Rafael silva
23,877 Pointssorry but this code doesn't work , the right code is like this :
for(i = 2 ; i <= 25; i += 2 ){ console.log(i); }

layeesolo
7,316 PointsThanks a lot.
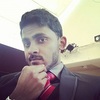
Sobin Sebastian
5,348 Pointsfor ( i = 2; i <= 24; i += 2 ) { console.log ( i ); }
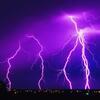
Janelle Mackenzie
7,510 PointsMost of you are correct, but you're missing the let statement for the 'i'.....
for ( let i=2 ; i<25 ; i+=2 ) {
console.log(i);
}

Abdi Nur
3,802 PointsJoseph yours works too, I think the %2 was mentioned in mdn
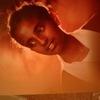
Million Asseghegn
3,845 Pointsvar eveni= [1, 2, 3, 4, 5, 6, 8, 9, 10]; for(i=0; i<eveni.length; i+=2){ if(i % 2===0){ console.log("even",i); }}

Starky Paulino
Front End Web Development Techdegree Student 6,398 Pointsfor ( var i = 2 ; i <= 24 ; i++ ) { if ( i % 2 === 0 ) { console.log (i);
}
}

Diane Bond
8,011 Pointsvar number = 0;
do{ number += 2; console.log(number);
}while(number< 24);

Stefan Riley
Front End Web Development Techdegree Graduate 13,099 PointsHi everyone,
I'm trying to submit this answer and I'm not sure if its being buggy or not. Could someone help me out?
for (i = 2; i <= 24; i += 2) { console.log(i);
Umar Ali
7,202 PointsUmar Ali
7,202 PointsUse 'let' to remove the scope from global
for ( let i = 2; i <= 24; i += 2) { console.log(i); }