Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial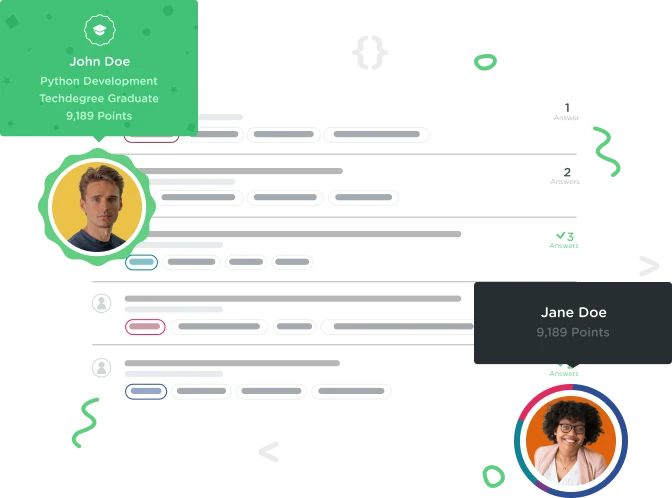

Natasha Sutevski
2,557 Pointslogging current array value to console...stuck
not sure when/if using .length might be useful.
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 100; i => 10; i -=1 ) {
console.log (temperatures[i]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
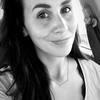
Christine Bogdan
11,349 PointsThe .length property tells you how many items are currently in an array. In your case, temperatures.length = 8, since there are 8 items (100,90,99,80,70,65,30,10) in the temperatures array.
I've corrected your code to:
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0; i < temperatures.length; i += 1 ) {
console.log (temperatures[i]);
}
Your code didn't run, because you set i = 10. In your code block however, you use console.log(array[i]). array[i] accesses a value at a certain index in an array.
So in order to be able to iterate through the array, you need to set var i = 0, to be able to start with the value at the zero index (meaning the first position in your array).
An overview of index and value in your array:
index 0 is value 100, index 1 is value 90, index 2 is value 99, index 3 is value 80, index 4 is value 70, index 5 is value 65, index 6 is value 30, index 7 is value 10.
With i = 0, you set array[i] to array[0], and you are then looking at the value at the zero index in your array (which is 100). If you use array[i] in console.log you will then print every value of the array into the console, with i = 0 being 100, i = 1 being 90 etc.
I hope this helps. :)

Kevin Gates
15,052 PointsMatthijs Boet 's answer was left as a comment instead of an "answer".
So you can more easily find it (or others if looking for this question with an answer), here is his response:
It can by useful if you want to know how many times you have to iterate over an array to loop over each item.
const temperatures = [100, 90 ,99 ,80 ,70 ,65 ,30 ,10];
for (let i= 0; i < temperatures.length; i +=1 ) {
console.log (temperatures[i]);
}
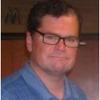
Mark Wilkowske
Courses Plus Student 18,131 PointsTry this and it will print out the array index number after the array element
{
console.log(temperatures[i], i);
}
100 0
90 1
99 2
80 3
70 4
65 5
30 6
10 7
Matthijs Boet
23,117 PointsMatthijs Boet
23,117 PointsIt can by useful if you want to know how many times you have to iterate over an array to loop over each item.
const temperatures = [100,90,99,80,70,65,30,10]; ​ for (let i= 0; i < temperatures.length; i +=1 ) { console.log (temperatures[i]); }