Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial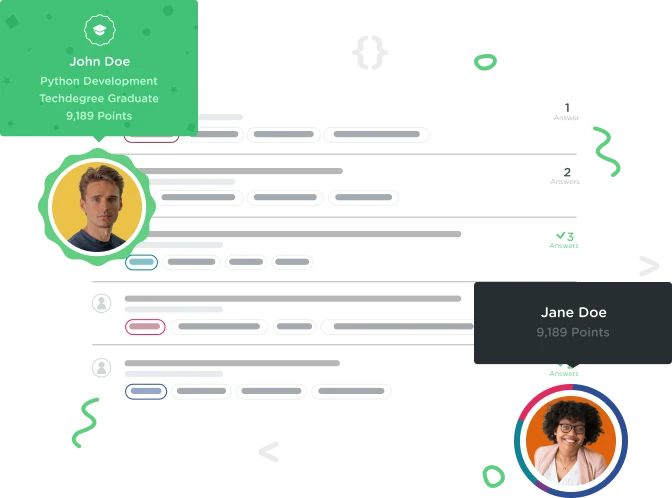
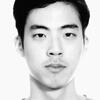
Harry Han
19,850 PointsLogging not working
I have the exact same code as Kenneth and yet I'm still able to see the 'X'. What am I doing wrong? I've tried running the code on other text editors and it's the same result. Calling game.log shows me that locations for monster, door, and player are logged, but has no affect in hiding it when the code is run.
import logging
import random
logging.basicConfig(filename='game.log', level=logging.DEBUG)
player = {'location': None, 'path': []}
cells = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(cells)
door = random.choice(cells)
start = random.choice(cells)
if monster == door or monster == start or door == start:
monster, door, start = get_locations()
return monster, door, start
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player in [(0, 0), (1, 0), (2, 0)]:
moves.remove('LEFT')
if player in [(0, 0), (0, 1), (0, 2)]:
moves.remove('UP')
if player in [(0, 2), (1, 2), (2, 2)]:
moves.remove('RIGHT')
if player in [(2, 0), (2, 1), (2, 2)]:
moves.remove('DOWN')
return moves
def move_player(player, move):
x, y = player['location']
player['path'].append((x, y))
if move == 'LEFT':
player['location'] = x, y - 1
elif move == 'UP':
player['location'] = x - 1, y
elif move == 'RIGHT':
player['location'] = x, y + 1
elif move == 'DOWN':
player['location'] = x + 1, y
return player
def draw_map():
print(' _ _ _')
tile = '|{}'
for idx, cell in enumerate(cells):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player['location']:
print(tile.format('X'), end='')
elif cell in player['path']:
print(tile.format('.'), end='')
else:
print(tile.format('_'), end='')
else:
if cell == player['location']:
print(tile.format('X|'))
elif cell in player['path']:
print(tile.format('.|'))
else:
print(tile.format('_|'))
monster, door, player['location'] = get_locations()
logging.info('monster: {}; door: {}; player: {}'.format(
monster, door, player['location']))
while True:
moves = get_moves(player['location'])
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player['location']))
draw_map()
print("\nYou can move {}".format(', '.join(moves)))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if not move in moves:
print("\n** Walls are hard! Stop running into them! **\n")
continue
player = move_player(player, move)
if player['location'] == door:
print("\n** You escaped! **\n")
break
elif player['location'] == monster:
print("\n** You got eaten! **\n")
break
else:
continue
1 Answer
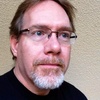
Chris Freeman
Treehouse Moderator 68,441 PointsGreat question!! On first glance I’m wondering why Kenneth’s code doesn’t produce an “X”. And I've finally figured it out.
TL;DR: Logging works, your code is fine. Kenneth's code in video has a bug (bad if
comparison)
In the video code, there is a bug in the draw_map
function. The blocks of code under the if
and else
sections are not the same. The if
block uses:
if cell == player['location']:
the else
block uses:
if cell == player:
The latter comparison will always fail because cell
is a tuple
and player
is a dict
.
In copying the code, you used the correct comparison to player['location']
.
Post back if you have more questions. Good luck!!
Riley Ittidecharchoti
3,195 PointsRiley Ittidecharchoti
3,195 Pointshad this bug been fixed in the code provided in the workspace? if not how to fix it? I was planning to study this code
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsIt appears the workspace file dd_game.py has the correction.