Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial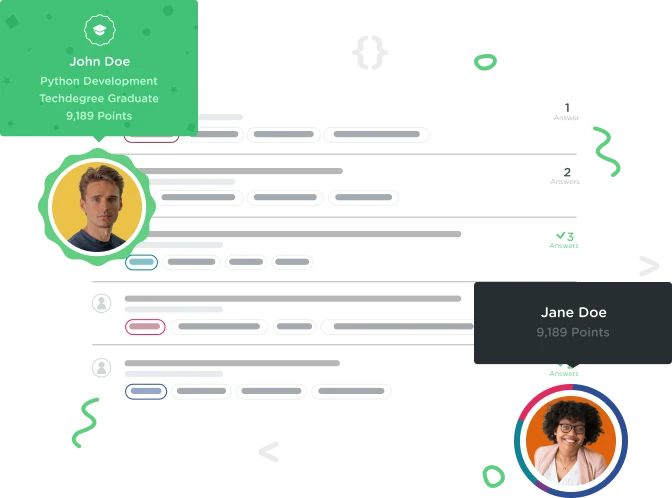
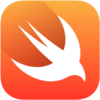
Antonija Pek
5,819 PointsLogic and syntax problem
// this function takes a string and prints it
func printString(input : String) {
println("Printing a string passed in: \(input)")
}
printString("A simple function")
/*
Assing the function we just declared
to a constant. NOTE that we do not add
parentheses "()" after the function name.
*/
let someFunction = printString
someFunction("Hi, look at me!")
func displayString(printStringFunc: (String) -> Void) {
printString("I am a function inside another function")
}
displayString(printString)
I don't understand how this is the output
Printing a string passed in: A simple function
Printing a string passed in: Hi, look at me!
Printing a string passed in: I am a function inside another function
Why is Printing a string passed in:
always first , before other strings? I watched this video 4 times and I am getting nowhere.
4 Answers
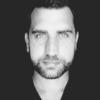
Jhoan Arango
14,575 PointsOk.. So we know that functions have parameters, they take an input of any type, it can be an Int, a Bool, or a string as long as you specify one kind of type it should take.
A function itself has a type. Letโs create a function and see what type it is.
func greeting(name: String)-> String {
return "Hello \(name)"
}
The type for the greeting function is (String) -> String.
Now we can create a function that in one of itโs parameter will take another function of type (String) -> String as input.
Example:
- func myFunction(stringFunc: (String) -> String, a: String, b: String) - > String {}
This function has 3 parameters. One is called stringFunc and is of type (String) -> String, the other one is called a, and is of type String, and the third parameter is b of type String. The comma is used to separate the parameters.
func myFunction(stringFunction:(String)-> String, a: String, b: String) -> String{
return "Hello \(a) and \(b)"
}
myFunction(greeting, a: "Jhoan", b: "Antonija")
// This will print โHello Jhoan and Antonija"
- Notice how the first parameter for myFunction is of the same type as the function greeting (String) -> String and its named is stringFunction, the second and third parameters which are named a, and b are of type String. These are used as the two input values for the provided greeting function.
Hope you understand a bit better. Read it over, and if you have questions let me know.
Good luck
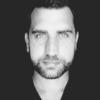
Jhoan Arango
14,575 PointsHello Antonija.. :
Ok, closures are a difficult part of swift, one that may take a little longer to understand. But what you are learning on this particular video is not a closure per say. You are learning about functions taking other functions as parameters. For these videos I suggest you read Function Types in the book The Swift Programing Language.
This will help you understand better, and will prepare you for closures.
Now, there will be a challenge coming up, that you may be a bit confused, but if you read the Function Types from that book, you may be able to solve it.
If you still need a deeper explanation about that challenge, click Here to go to an explanation I gave someone who was confused about it too.
Hope this answer helps you to be guided to the right direction, and if you still have more questions you can tag me on it @jhoanarango
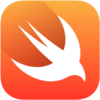
Antonija Pek
5,819 PointsI have read it but Apple's explanations are to hard for me. I have read your answer and I still can't find the answer for this:
func mathOperation(anyAddFunc: (Int, Int) -> Int, a: Int, b: Int) -> Int{
let me shorten that:
(anyAddFunc: (Int, Int) -> Int, a: Int, b: Int)
This is the first time I see , (a coma) and then names of variables and then type. This confuses me a lot. How do I know when to write this, when not. There is so much return arrows and parentheses.
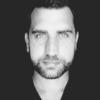
Jhoan Arango
14,575 PointsWorking on your answer....
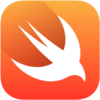
Antonija Pek
5,819 PointsHi, thanks for providing a detailed answer. It is somewhat clearer to me. I am still struggling with a (String) -> String
but hopefully I will understand that soon.