Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial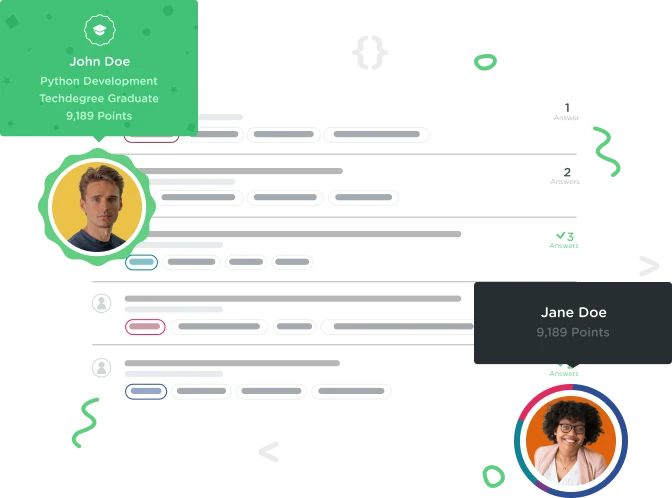
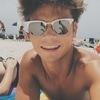
garyguermantokman
21,097 PointsLogic Only (tutorials?), Swift help with checking for vowels and constants! (no personal code)
I am trying to check string for vowels and consonants in Swift ?
example : "Hi there" = 3 constants and 3 vowels
1 Answer

james white
78,399 PointsHi Gary,
I'm guessing you might mean 'consonants' (not 'constants')?
You didn't mention if your strings are going to be confined (or validated) to only be lower case.
If you need the ".lowercase" function in "pre-preparing" your input string
you may need to use 'import Foundation' as this StackOverFlow thread mentions:
However (being somewhat lazy) I just copied and pasted your question into google
and one of the first results that came up was this StackOverFlow thread:
http://stackoverflow.com/questions/31954013/constants-and-vowels-swift
Also at the top of the Google results was this tutorial page:
https://code.csdn.net/theSalt/swift/file/Functions.md
...which had this code:
func count(string: String) ->
(vowels: Int, consonants: Int,
others: Int) {
var vowels = 0, consonants =
0, others = 0
for character in string {
switch
String(character).lowercaseString {
case "a", "e", "i",
"o", "u":
++vowels
case "b", "c", "d",
"f", "g", "h", "j", "k", "l",
"m",
"n", "p", "q", "r",
"s", "t", "v", "w", "x", "y",
"z":
++consonants
default:
++others
}
}
return (vowels, consonants,
others)
}
It even provides some example code for the use of the count function:
let total =
count("some arbitrary string!")
println("(total.vowels) vowels and (total.consonants) consonants")
// prints "6 vowels and 13 consonants"
By the way - the reason it might return 'others' is a string could contain punctuation marks
or other extended ASCII characters above 127:
There is also some 'func count" code on this page
(that says the code is excerpted from Apple documentation):
http://www.techiespoint.com/1998/function-declaration-swift
In fact, looking at the Swift docs:
I even managed to find this code:
let someCharacter: Character = "e"
switch someCharacter {
case "a", "e", "i", "o", "u":
print("\(someCharacter) is a vowel")
case "b", "c", "d", "f", "g", "h", "j", "k", "l", "m",
"n", "p", "q", "r", "s", "t", "v", "w", "x", "y", "z":
print("\(someCharacter) is a consonant")
default:
print("\(someCharacter) is not a vowel or a consonant")
}
// prints "e is a vowel"
I believe Swift even has extensions that can add new instance and type methods to existing types,
(including add nested types to existing types).
So code related to what you are asking for might be:
extension Character {
// nested type
enum Kind {
case Vowel, Consonant, Other
}
// computed property
var kind: Kind {
switch String(self).lowercaseString {
case "a", "e", "i", "o", "u": return .Vowel
case /* consonant */: return .Consonant
default: return .Other
}
}
}
var char: Character = "c"
char.kind // Character.Kind.Consonant
--the above code comes from Section 20 on this page:
http://www.blaenkdenum.com/notes/swift/
Hopefully that's enough to get you started...