Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial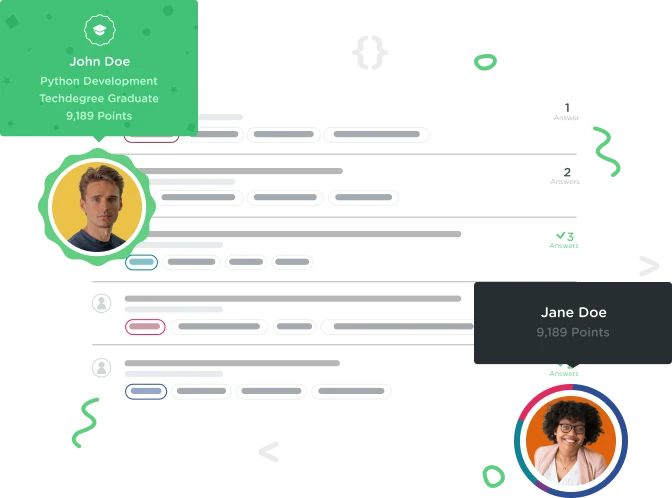
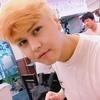
Gong T
1,891 PointsLOGICAL OR is not working in "answerxx" variable
Hello, I just finished typing up my code for "The Conditional Challenge". Since the answers to the questions that will appear in the "prompt" could be either lowercase or uppercase I am trying to implement the Logical OR, but the program I wrote is only taking the answers in lowercase as correct answers...while ignoring uppercase answers. For example, if the prompt asks "What is the color of an apple?", it will only accept "red" as the correct answer and doesn't log "Red" as the correct answer.
The following is my codes with comments. What would you recommend? Should I try something different like the && operator?:
let correctAnswer = 0;
//Questions that will be asked.
const questionOne = prompt(`1. What is the color of an apple?`);
const questionTwo = prompt(`2. What is the color of an orange?`);
const questionThree = prompt(`3. What is the color of a grape?`);
const questionFour = prompt(`4. What is the color of a banana?`);
const questionFive = prompt(`5. What is the color of kiwi?`);
//Answers to the questions in the prompt
const answerOne = "red" || "Red";
const answerTwo = "orange" || "Orange";
const answerThree = "purple" || "Purple";
const answerFour = "yellow" || "Yellow";
const answerFive = "green" || "Green";
//One point will be added to each correct answer.
if ( questionOne == answerOne ) {
correctAnswer += 1;
}
if ( questionTwo == answerTwo ) {
correctAnswer += 1;
}
if ( questionThree == answerThree ) {
correctAnswer += 1;
}
if ( questionFour == answerFour ) {
correctAnswer += 1;
}
if ( questionFive == answerFive ) {
correctAnswer += 1;
}
//All the total correct answers will be added and final ranking results will display in main element.
if ( correctAnswer >= 5 ) {
document.querySelector('main').innerHTML = `CONGRATS! You got all the answers correct! You are GOLD!`;
}
else if ( correctAnswer == 3 || correctAnswer == 4 ) {
document.querySelector('main').innerHTML = `You almost got all of them correct! You are SILVER!`;
}
else if (correctAnswer == 1 || correctAnswer == 2 ) {
document.querySelector('main').innerHTML = `Not that bad! You got most incorrect! It's okay! You get Bronze!`;
} else {
document.querySelector('main').innerHTML = `Not good at all....you got all the answers incorrect!`;
}
Thank you for taking time out of your busy day to review this! I appreciate it!
2 Answers
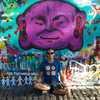
Andrew Stelmach
12,583 PointsThe logic is incorrect eg const answerOne = "red" || "Red";
const answerOne = "red" || "Red";
'red' === answerOne // this will return true
'Red' === answerOne // this will return false
This is because "the || operator actually returns the value of one of the specified operands, so if this operator is used with non-Boolean values, it will return a non-Boolean value." (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_OR)
const answerOne = "red" || "Red";
evaluates to "red"
. I expect this statement evaluates to the first truthy value, which is "red"
.
So when you do "Red" === answerOne;
you will get false
because "Red" is not equal to the value of answerOne
which is "red"
.
There are probably several ways to solve your problem but I would probably do:
...
const answerOne = 'red';
...
if (questionOne.toLowerCase() === answerOne ) {
correctAnswer += 1;
}
...
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/toLowerCase
Note it's generally good practice to use the strict equality operator - ===
(as I have above) - rather than the ==
you're using here, unless you actually need the flexibility of the ==
operator https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Equality
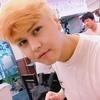
Gong T
1,891 PointsAndrew Stelmach Thank you so much! I understand how to properly use the .toUpperCase()
and ===
now! You are awesome! Hope you have a great day and week!
Gong T
1,891 PointsGong T
1,891 PointsHi Andrew! Thank you so much for your review and answers!! I rewrote the whole code and found an even better way to write this program. Please let me know what you think. Thank you!
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 PointsI'm not familiar with the specific task you're working on, but yeah that latest version of your code using
.toUpperCase()
and the===
operator looks good to me Gong T