Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial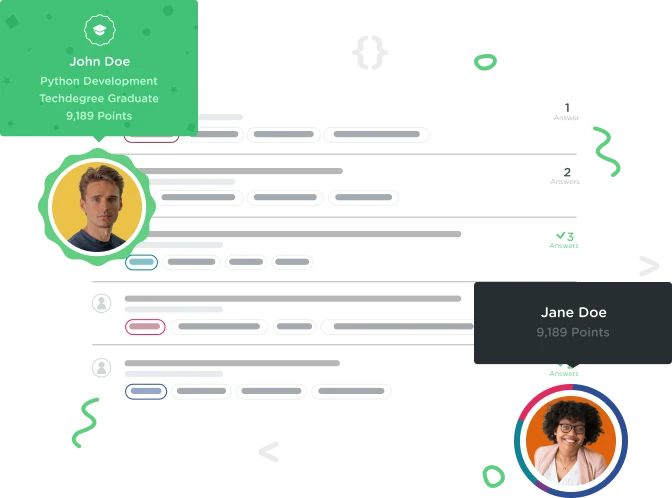

orange sky
Front End Web Development Techdegree Student 4,945 PointslogTeams function
Hello, In the Portland object from the lecture video, there are 2 methods, and one of them is logTeams. Is logTeams an anonymous function? I guess what I am trying to say is: Are all methods created in an object literal (like Portland) anonymous? Or is the key or property name also the name of the function ( ie. logTeams is the name of the key or property ). Please look at the video. you will see what I mean: logTeams : function () { ...}
2 Answers
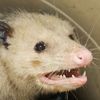
gregsmith5
32,615 Points"Is logTeams an anonymous function?"
Yes!
"Are all methods created in an object literal (like Portland) anonymous?"
Not necessarily!
"Or is the key or property name also the name of the function"
No!
JavaScript compilation is a hairy topic that's very easy to get wrong, so I won't pretend to be qualified enough to give you a full answer. I can give you the "What you need to know" version, though.
Functions in JavaScript are objects. They have properties just like any other object you've seen (including those in the referenced video). One such property is name. Essentially, functions with this property set to an empty string are anonymous functions, while those with the property defined are not. Check this out:
function notAnonFunction () {
//...
}
console.log(notAnonFunction.name); //"notAnonFunction"
var someAnonFunction = function() {/*...*/};
console.log(someAnonFunction.name); // ""
var notAnonFunctionExpression = function helloThere() { /*...*/ }; //Variable name has nothing to do with function name
console.log(notAnonFunctionExpression.name); // "helloThere"
// This holds true inside of object literals
var someObject = {
prop1: function() {/* This is an anonymous function */ }, //Property name has nothing to do with function name
prop2: function coffeeIsGreat() {/* This is not an anonymous function */}
}
// Named functions can be called recursively
var hero = function zapRowsdower() {
if(beer) {
zapRowsdower();
}
}
Hope this helps!

John Fox
Courses Plus Student 2,370 PointsIn the video, logTeams
is initially an anonymous function.
logTeams: function() {
console.log(this.soccerTeams);
}
(05:40) - But later Huston says:
I'm actually going to move my logTeams function down here to its own anonymous function.
And then he writes:
function logTeams () {
console.log(this.soccerTeams);
}
I thought the above code is a function declaration not an anonymous function???
(06:15) - Or, is Huston referring to the below code as the function expression? Isn't it just a function being assigned as a value in the Portland object?
Portland.foo = logTeams;
John Fox
Courses Plus Student 2,370 PointsJohn Fox
Courses Plus Student 2,370 PointsIsn't this actually a function expression?
MDN Docs
Or am I misunderstanding named function expressions?