Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial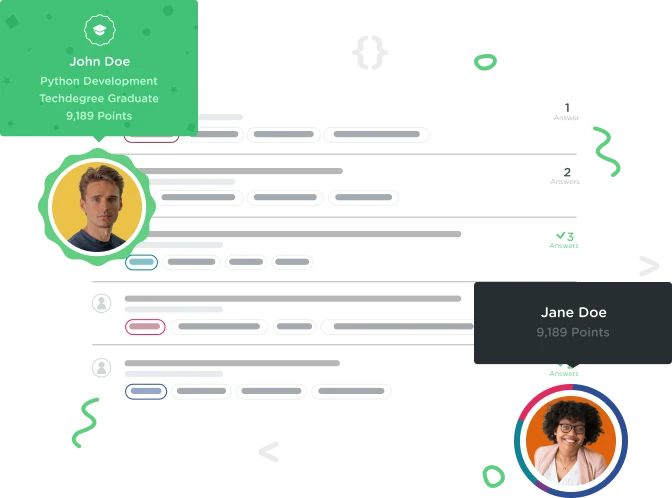

Martins Rokis
6,995 Pointslooking for error
hi, i am so close to finish this course, but in final step i got message "I expected to see the names I passed in through the args, but I do not. Hmm." which arguments are expected? please help thanks
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
public ForumPost(User author, String title, String description ){
mAuthor=author;
mTitle=title;
mDescription=description;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
public User(String firstName, String lastName) {
mFirstName=firstName;
mLastName=lastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic){
mTopic=topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("Craig","Dennis");
ForumPost post = new ForumPost(author,"Java","Craig");
forum.addPost(post);
}
}

Martins Rokis
6,995 Pointsi am confused which values are expected in ForumPost post = new ForumPost(); can somebody show me please, i can't solve it i think first argument is ForumPost post = new ForumPost(author,?,?); but then i need 2 strings title and description, where are those?

alex novickis
34,894 PointsYes it is confusing a bit,
the main is called by something in the treehouse test system that passes that to you they are passed in command line arguments to the main - the only hint they give you is the example in the comment of the challenge
it looks like they give you this set of stuff in the args --> "java Example Craig Dennis"
so args[0] would be "java", and args[1] would be "Example", args[2] would be "Craig", and args[3] would be "Dennis"
So you'll have to use those variables when creating the new forum, author, and post
So for example in Forum object, you can do something like
Forum forum = new Forum(args[0])
Now you have to do the other 2 objects with the correct args[]
alex novickis
34,894 Pointsalex novickis
34,894 Pointssomething is being passed in here the args here on this line public static void main(String[] args)
the example is "java Example Craig Dennis"
so you will need to take args string array apart and pass along the correct pieces to all the other functions
Right now your code is using values you put in as "Java", "Craig", "Dennis", etc