Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial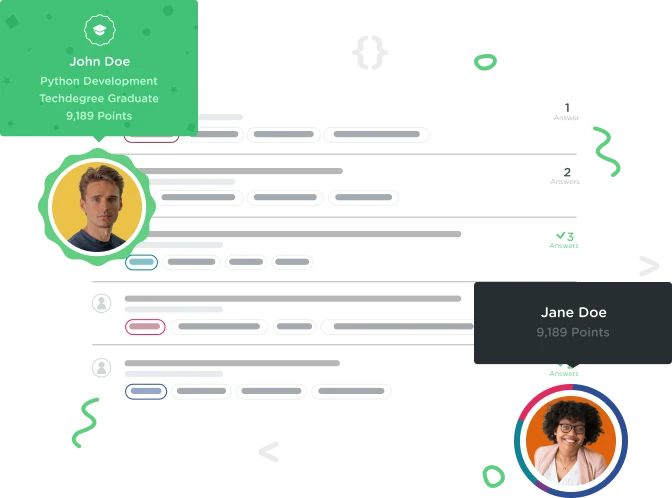

Chris Utley
9,300 PointsLooking for feedback on my code's efficiency, please and thank you! :)
alert('Welcome to the random number generator, pick a number.');
//User prompted to enter numbers
var num1 = parseInt(prompt('First Number: '));
var num2 = parseInt(prompt('Second Number: '));
//Random Number Generator
function userNum(num1, num2){
//Check which number is larger before calculating
if (num1 > num2) { var randNum = Math.floor(Math.random() * (num1 - num2 + 1) + num2); return randNum; }
else { var randNum = Math.floor(Math.random() * (num2 - num1 + 1) + num1); return randNum; }
}
//Return random number to webpage
document.write('<h1>Your random number is ' + userNum(num1, num2) + '.</h1>');
1 Answer
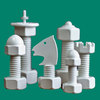
Steven Parker
231,275 PointsYou program is perfectly functional as-is, good job! But here's a couple of improvement suggestions:
You can make the code a bit more "DRY" by creating a function to generate the random number (like the one shown in the next video), and calling it from inside your other function instead of duplicating the formula.
And you can avoid creating the "randNum" variable by directly returning the formula result (or the returned value from a new random function).