Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial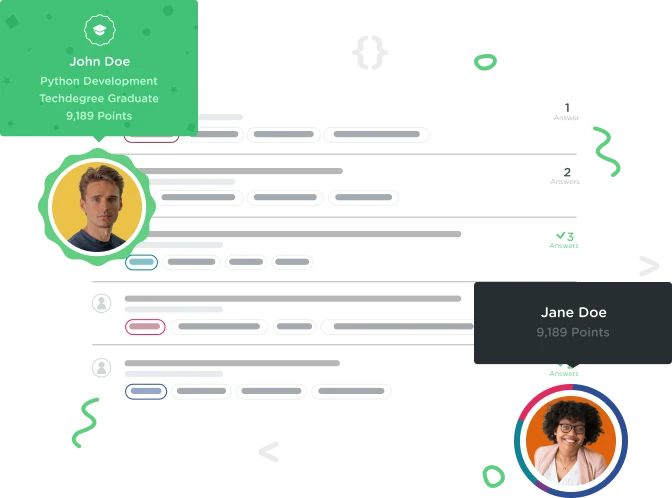
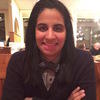
Olivia Orr
7,921 PointsLooking for feedback RE: printing results to the page
I can't help but feel like there's a much easier way to go about printing the results of my quiz to the page. Please feel free to chime in with any insight you might have. I almost gave up on this challenge, but I finally figured it out today - I'm happy to hear any other comments/suggestions not related to the printing as well. Thanks in advance!
//Create a 2D array with questions and answers
var harryPotterQuiz = [
["What was the name of Harry's owl?", "hedwig"],
["What was the name of Hagrid's dog?", "fang"],
["What was the name of Hagrid's pet spider?", "aragog"],
["What's the name of the Killing Curse?", "avada kedavra"],
["What is Professor Dumbledore's first name?", "albus"],
["What magical plant gives Harry gills during the Triwizard Tournament?", "gillyweed"]
];
//Create a function that prints a message to the screen
function print(message) {
document.write("<p>" + message + "</p>");
}
//Create a function that prints a message with number of correct guesses
function printScore() {
var scoreHTML = "<h3>" + "Congrats! You answered ";
scoreHTML += guessCorrect.length + " out of ";
scoreHTML += (guessCorrect.length + guessIncorrect.length) + " questions correctly!";
scoreHTML += "</h3>";
print(scoreHTML);
}
//Create a function that prints a list of correctly answered and incorrectly answered questions
function printFinished(answerList) {
var listHTML = "<ul>";
for (var counter = 0; counter < answerList.length; counter += 1) {
listHTML += "<li>" + answerList[counter] + "</li>";
}
listHTML += "</ul>";
print(listHTML);
}
//Create two arrays that store the incorrectly/correctly answered questions
var guessCorrect = [];
var guessIncorrect = [];
//Create a function that prompts the user to answer the questions
function startQuiz(quizList) {
for (var i = 0; i < quizList.length; i += 1) {
var question = prompt(quizList[i][0]);
question = question.toLowerCase();
if (question == quizList[i][1]) {
guessCorrect.push(quizList[i][0]);
} else {
guessIncorrect.push(quizList[i][0]);
}
}
}
//Start the quiz
startQuiz(harryPotterQuiz);
//Print the score
printScore();
//Print the correct/incorrect questions - if no questions are answered correctly or incorrectly, the text is not displayed
if (guessCorrect.length > 0) {
print("You answered these questions correctly:");
printFinished(guessCorrect);
}
if (guessIncorrect.length > 0) {
print("You answered these questions incorrectly:");
printFinished(guessIncorrect);
}
1 Answer
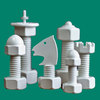
Steven Parker
230,274 PointsYou will learn much better methods later.
The focus here is the programming. The input and output methods used here are not things you would commonly see in actual practice except for testing. In other courses you'll learn much more sophisticated methods of interacting with the user through form elements, styling, and DOM manipulation. So don't worry about refining the output now.
But your code looks fine, from the perspective of this course. Good job.