Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial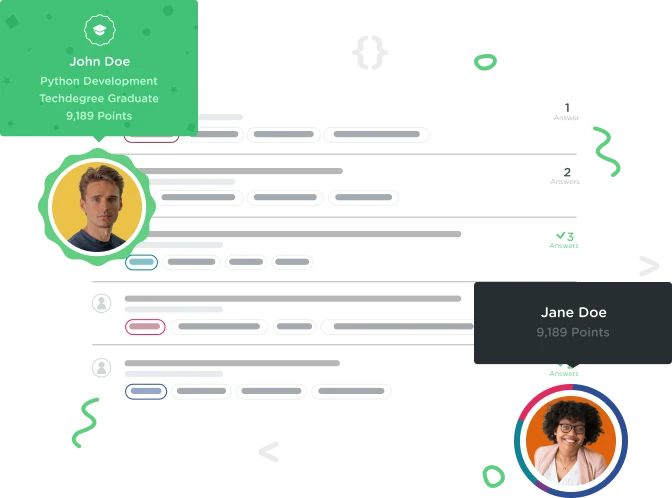

Jae Min Kweon
4,695 PointsLooking for possible improvements!
I have a working code for the additional challenge, but felt like there might be some room for improvement. I've marked some particular sections with comments pointing out my question, but any and all advice are welcome!
var students = [
{ name: 'Albert', track: 'iOS', achievements: 100, points: 12000 },
{ name: 'Betty', track: 'Java', achievements: 120, points: 12600 },
{ name: 'Charlotte', track: 'JavaScript', achievements: 420, points: 46000 },
{ name: 'Dennis', track: 'Perl', achievements: 220, points: 25000 },
{ name: 'Elijah', track: 'Python', achievements: 300, points: 34200 },
{ name: 'Elijah', track: 'HTML', achievements: 240, points: 28000 }
];
var searchName = ''; //empty string to save search names to.
var result = ''; //empty string to save search results to.
var resultCount = 0; //variable to hold the number of results yielded by the given name
function print(string) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = string;
}
/*
The 'retriever' function sets up the string to be printed out as html.
It is a bit different from the function in the example script. I tried to utilize the 'for in'.
I am still unsure if declaring the local variable 'html' separately is necessary, just did it habitually.
Was curious if I could just declare it in the code block under 'if'.
*/
function retriever(array) {
var html = '';
for (var prop in array) {
if (prop === 'name') {
html += '<h2>student: ' + array[prop] + '</h2>'
} else {
html += '<p>' + prop + ': ' + array[prop] + '</p>'
}
}
return html
}
while (true) {
searchName = prompt('Please enter a student name.');
if (searchName === null || searchName.toUpperCase() === 'QUIT') {
break;
}
else {
for (var i = 0; i < students.length; i += 1) {
if (searchName === students[i].name) {
result += retriever(students[i]);
resultCount += 1
}
}
//An alert when there is no matching name.
if (resultCount === 0) {
alert('Matching name not found in database.')
}
//An alert when there is a matching name.
else {
alert(resultCount + ' result(s) were found!')
print(result);
}
result = ' ';
resultCount = 0;
/*
I am using brute-force method to set the result and resultCount variable back to its original value,
in order to clear the results from the current search so that it won't collide with results from newer search
I wonder if there would be any better approach then this, because I felt like there should be a better way.
*/
}
}
1 Answer

Josh Thomas
8,019 PointsI'm new to programming and I am learning a lot in these courses. I really liked your approach, opened my mind to some different ways to do things! Thanks!
Jae Min Kweon
4,695 PointsJae Min Kweon
4,695 PointsGlad to know that my approach was helpful for you!