Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial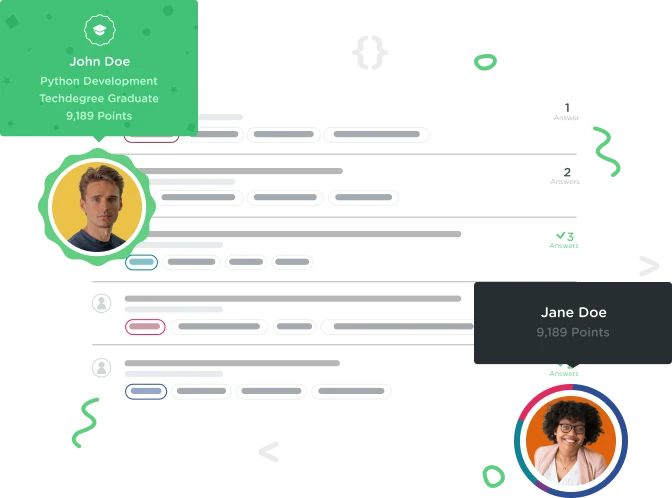
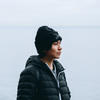
Zhenghao He
Courses Plus Student 2,389 PointsLooking for ways to adding css animation to random quote generator
Hi Guys,
I'm working on the first project of full stack techdegree, which is to build a random quote generator. It has a button and each time it will show a different quote with random background color.
I am wondering if there's ways to improve this project by adding more animation effect.
I image that I change the background to the portrait according to every quote and make the portrait slide out and in each time I press the button.
I was experimenting with jQuery to add a animation class to the portrait to achieve that effect, but I couldn't seem to have the first image slide from the left to the center and slide to the right and disappear after the second image comes. I know it sounds confusing but the effect I'm trying to get here is like a sequential fashion of sliding in and out each time the button is clicked.
Could you guys give me some hints?
And another thing is to add portraits, do you think it is better to change the body's background image or add new img tag?
Thanks
---update--- my work is at https://github.com/zhenghaohe/random-quote-generator There is a live demo link you guys can try. I now right now it doesn't look super interesting.
first of all I found it so hard to crop my picture to fit the screen. This is how I add the pictures of each artists
background-image: url("../img/Migos.jpg");
background-size: cover;
secondly the way I change the background is to set a class for each artist and toggle it when the button is pressed
.migos {
background-image: url("../img/Migos.jpg");
background-size: cover;
-webkit-animation: slide 25s linear infinite;
}
.lilPump {
background-image: url("../img/pump.jpg");
background-size: cover;
-webkit-animation: slide 25s linear infinite;
}
.bigShaq {
background-image: url("../img/bigshaq.jpg");
background-size: cover;
-webkit-animation: slide 25s linear infinite;
}
since I use classed to change the background, I'll have to remove the previous class before I add any new ones, that means I have to keep track of the last generated quote object
var getRandomQuote = function() {
last = viewedQuotes[viewedQuotes.length-1];
var random = Math.floor(Math.random() * (quotes.length));
quote = quotes.splice(random,1)[0];
if (quotes.length == 0) {
quotes = viewedQuotes;
viewedQuotes = [];
}
viewedQuotes.push(quote);
return [quote,last];
};
function printQuote() {
arr = getRandomQuote();
quote = arr[0];
last = arr[1];
message = '<p class="quote">' + quote.quote + '</p>' + '<p class="source">'+ quote.source;
document.getElementById('quote-box').innerHTML = message;
var body = $('body');
$('body').addClass(quote.rapper);
$('body').removeClass(last.rapper);
}
Now it works fine but I noticed there was an error in console that
Uncaught TypeError: Cannot read property 'rapper' of undefined
at HTMLButtonElement.printQuote (script.js:67)
I guess it's because at the first the there is no previous quote object, so
$('body').removeClass(last.rapper);
caused this error. but I cannot think of any way to prevent this . I tries use
if (last.rapper !== undefined) {
$('body').removeClass(last.rapper);
}
to prevent accessing it for the first time but I still got the error.
1 Answer
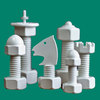
Steven Parker
229,786 PointsI was just fiddling around and came up with this, using plain JavaScript and CSS transitions:
<div class="box">
<div class="msg"></div>
</div>
<button class="run">Animate!</button>
div {
height: 300px;
width: 300px;
transition: transform 1s, background-color 2s 1s, color 3s 1s;
overflow: hidden;
}
.msg {
display: table-cell;
background: skyblue;
font-size: 6em;
text-align: center;
vertical-align: middle;
transform: translateX(-100%);
}
document.querySelector(".run").addEventListener("click", e => {
var msg = document.querySelector(".msg");
var btn = e.target;
btn.disabled = true;
msg.parentNode.style.visibility = "visible";
msg.textContent = "Hello.";
msg.style.transform = "translateX(0)";
msg.style.color = "white";
msg.style.backgroundColor = "firebrick";
setTimeout( _=> {
msg.textContent = "Bye!";
setTimeout( _=> {
msg.style.transform = "translateX(100%)";
setTimeout( _=> {
msg.style.transition = "none";
msg.parentNode.style.visibility = "hidden";
msg.style.backgroundColor = "";
msg.style.color = "";
msg.style.transform = "";
setTimeout( _=> {
msg.style.transition = "";
btn.disabled = false;
}, 10);
}, 1000);
}, 1000);
}, 2000);
});
You can do even fancier things with CSS and/or jQuery animations.
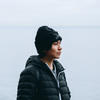
Zhenghao He
Courses Plus Student 2,389 Pointswow! your solution is great! the app I'm trying to build roughly looks like this https://zhenghaohe.github.io/random-quote-generator/ This is my work and I know it is flawed. Still trying to improve
Steven Parker
229,786 PointsSteven Parker
229,786 PointsPlease post the code you have now to provide a starting point.