Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial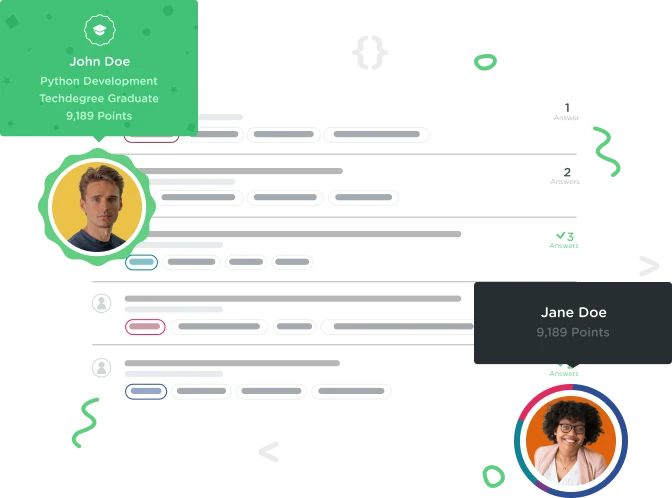

Colin Sandlin
4,512 PointsLooks like it prints the code instead of the correct content
// Define Variables //
var quizContent =
[
["What day of the month were you born?", "13"],
["In what month were you born?", "April"],
["Where were you born?", "Memphis"],
["Where did you grow up?", "Birmingham"],
["Where did you go to college?", "Auburn"]
];
var questions;
var answers;
var userResponse;
var userScore = 0;
var rightAnswers = [];
var wrongAnswers = [];
var html;
// Define print function (reused from last project)//
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// Function to begin to build content on page of which questions were right and wrong //
function pageContent(x) {
var writeHTMLtoPage = '<ol>';
for (var i = 0; i < x.length; i++) {
writeHTMLtoPage += '<li>' + x[i] + '</li>';
}
writeHTMLtoPage += '</ol>';
return writeHTMLtoPage;
}
//Turn for loop into function //
// Begin for loop that runs through each item of "quizContent" //
function runTheQuiz(thisQuiz) {
for (var i = 0; i < thisQuiz.length; i++) {
questions = thisQuiz[i][0]; // define questions as the first position of 2D array
answers = thisQuiz[i][1]; // define answers as the second position of 2D array
userResponse = prompt(questions);
if (userResponse === answers) {
userScore += 1;
rightAnswers.push(thisQuiz[i][0]); // if correct, add question item to correctAnswers array //
}
else {
wrongAnswers.push(thisQuiz[i][0]); // if wrong, add question item to wrongAnswers array //
}
}
}
runTheQuiz(quizContent);
html = "You got " + userScore + " out of 5 questions correct.";
html += '<h2>You got these questions correct:</h2>';
html += pageContent(rightAnswers);
html += '<h2>You got these questions incorrect:</h2>';
html += pageContent(wrongAnswers);
print(pageContent);
It prints this to the page, I have gone over the code for two hours and can't figure it out:
function pageContent(x) { var writeHTMLtoPage = ' '; for (var i = 0; i < x.length; i++) { writeHTMLtoPage += ' ' + x[i] + ' '; } writeHTMLtoPage += ' '; return writeHTMLtoPage; }
1 Answer

Colin Sandlin
4,512 PointsSorry, I figured it out right after I posted. The last line should say:
print(html);
instead of
print(pageContent);