Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial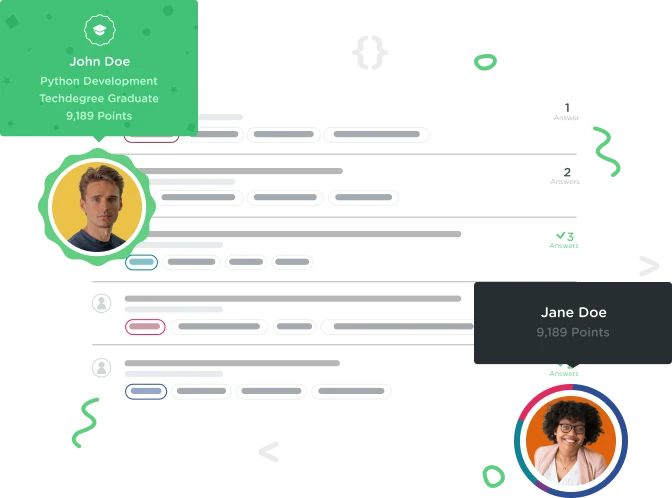

Michael Pastran
4,727 PointsLoop Challenge
No clue what i am doing wrong here. the only thing i can think of is that i am not incrementing it by 1 correctly each time that the loop runs. which is why its never going higher than 1? idk
numbers = []
number = 0
loop do
numbers = [number.to_i] + 1
if numbers.length > 3
break
end
end
3 Answers

Michael Pastran
4,727 PointsHey Gavin i finally got it!!
numbers = []
number = 0
loop do
if numbers.count >= 3
break
end
numbers.push(number)
number += 1
end
the thing is that the instructions are not clear that it has to equal or greater than 3. thank you tho!!

Gavin Ralston
28,770 PointsKeep up the good work!

Michael Pastran
4,727 PointsHey Gavin, do you know if there are any websites or books or anything really that has like different exercises and then displays the correct code/ variations to run the program. so pretty much like the challenges on TreeHouse, but something that gives you the exercise, you build the code and then compare it to the possible solutions for that exercise. I feel like something like that would be extremely helpful since i have noticed that i tend to forget some things since i don't get much practical practice of the concepts. (good idea for an app btw haha)

Gavin Ralston
28,770 PointsWell, one of the things I'm seeing is that you walk into the loop and the very first thing you do is try to add an integer to an array, which is causing your error message.
Have you been through the videos where it teaches you array methods like push?
numbers = []
numbers.push(3)
numbers.push("7".to_i)
Something like that, where you're adding an integer, or converting a string an actual integer and then adding it to an array, should work.

Michael Pastran
4,727 Pointsnot really. i mean to be honest Jason talked about it but only briefly to say that its a way to add an item to an Array. we also used it in the last video where we create the grocery list program. but there it was used to add a HASH into an ARRAY. let me see if i can figure it out using the .push method. Thanks Gavin

Gavin Ralston
28,770 PointsTo be more clear, what I meant was:
"You start out by actually trying to add a collection to a number" instead of trying to put that number in the array. :)
I realize you're actually trying to add a number to an array, so what I said might seem silly. :) One way is to #push it on to an array.
Rather than using +, which will try to literally to add the two things together, kind of like saying "What is 3 plus a handful?"
handful = [1, 2]
handful = handful + 3 # not doing what you think it's doing
you want to be saying "Put 3 into the handful." That's what push will do.
handful.push(3) # doing what you probably want to be doing

Michael Pastran
4,727 PointsUsing the loop construct, add the current value of number to the numbers array. Inside of the loop, add 1 to the number variable. If the numbers array has more than 3 items, use the break keyword to exit the loop................. those are the instructions. i still cant figure it out. the part that im stuck in is the adding 1 to the number variable. im assuming that every time the loop runs it will add 1 more to the value. so it will be 0 , 1 , 1 . and at this point it will break because of our condition??
numbers = []
number = 0
this is the code that i come up with.
numbers = []
number = 0
loop do
if numbers.length > 3
break
end
numbers =[number.push(1)]
end
i thought i would put the addition at the bottom, since it will loop once and then check if the condition meets right? lol so confused with this one. sorry

Gavin Ralston
28,770 PointsThere are about a million ways to do things in ruby, so it can be hard to kind of get the hang of it.
A few ways to do this (and probably NOT where you're at in the tutorial series...) is like so:
3.times do
numbers.push(number += 1)
end
# or maybe
(1..3).each { |n| numbers.push(n) }
# or maybe
1.upto 3 do |num|
numbers.push(num)
end
etc, etc...
The key here isn't to necessarily understand what I'm doing anywhere else (although 1 and 3 should make a little sense) but look at what I'm doing in the middle of them all.
numbers.push(num)
numbers is an array. You established that right away when you said "numbers = []"
So you don't need to use brackets again for anything in this exercise. In fact, using them again can lead to all kinds of errors.
So from the moment you type numbers=[] you can now use any array method at your disposal, including push to get your job done.
So when you step into your loop, all you really need to do (and you were doing it fine, updating the number to add to the array every time through the loop) is just get the number put into the array.
so...
loop do
if you added enough numbers
break
#otherwise this will happen
push num to numbers array
end
Michael Pastran
4,727 PointsMichael Pastran
4,727 PointsTypeError: no implicit conversion of Fixnum into Array ................ this is the error that i am getting. from the error i think the issues is that i am putting an Integer into an Array....... numbers = [number] however i have no idea on how to change that. do i need to change the array into an Int? since the IF condition is checking to see if the length > 3 (3 being an Int).