Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial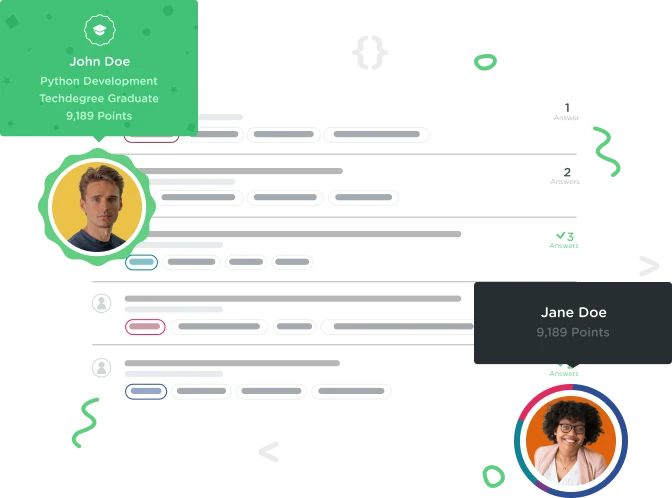
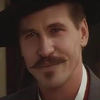
huckleberry
14,636 PointsLoop for an Array: What is this undefined I'm getting?
Hello Lovelies,
I'm doing a quick brush up of my studies from last night which went from loops -> Arrays and I just attempted a loop after another loop.
var drinks = ["coffee", "orange juice", "milk", "iced tea", "machiatto"];
for (var i = 0; i < 5; i+= 1){
console.log(drinks[i]);
}
console.log("2nd verse, same but reversed!");
for ( ; i >= 0; i -= 1){
console.log(drinks[i]);
}
And it works, but I'm getting an error after the console.log("2nd verse, same but reversed!");
part of the code that says undefined
.
Is this because I never defined the variable in the first expression within the 2nd for()
?
I know that the expressions within the ()
of the for()
are optional and assumed that, because I'm simply calling up the same variable that had been created in the previous loop, that I wouldn't need to redeclare it or initialize it within the first expression because that's optional and it has already been declared.
The first loop ended with i
's value being equal to 5. So, by starting the next loop, I figured I would not have to initialize by setting i
again.
So what is going on within the code that's is causing the undefined
?
Thanks!
2 Answers
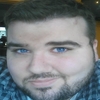
Marcus Parsons
15,719 PointsHey huckleberry,
The problem you're running into is that after that first for loop executes, "i" becomes 5. So when the 2nd loop starts, it is starting from an undefined index of 5. Only 0-4 are defined since you have 5 strings in the array. For that 2nd for loop, you'll have to explicitly set "i" again, and to make sure that these for loops are dynamic, I set "i" to be "drinks.length - 1" so that it will be in the correct index when it starts regardless of how many drinks you had to the "drinks" array:
var drinks = ["coffee", "orange juice", "milk", "iced tea", "machiatto"];
for (i = 0; i < drinks.length; i += 1){
console.log(drinks[i]);
}
console.log("2nd verse, same but reversed!");
for ( i = drinks.length - 1; i >= 0; i -= 1){
console.log(drinks[i]);
}

johnweinshel
Courses Plus Student 61 PointsMarcus' suggestion makes sense; I'd prefer to use a different counter, just for readability:
var drinks = ["coffee", "orange juice", "milk", "iced tea", "machiatto"];
for (var i = 0; i < 5; i++){
console.log(drinks[i]);
}
console.log("2nd verse, same but reversed!");
for ( var j = drinks.length - 1 ; j >= 0; j--){
console.log(drinks[j]);
}
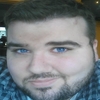
Marcus Parsons
15,719 PointsAbsolutely. I was just addressing what he was talking about. I also edited your code so that it will render properly. When you post code, it will render best if you wrap within 3 backticks like so:
huckleberry
14,636 Pointshuckleberry
14,636 PointsAhhhh ... I gotcha I gotcha. w00t, my first "off by one" error! Fantastic.
Thanks a bunch bro!
Cheers,
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsMy pleasure my treasure!