Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial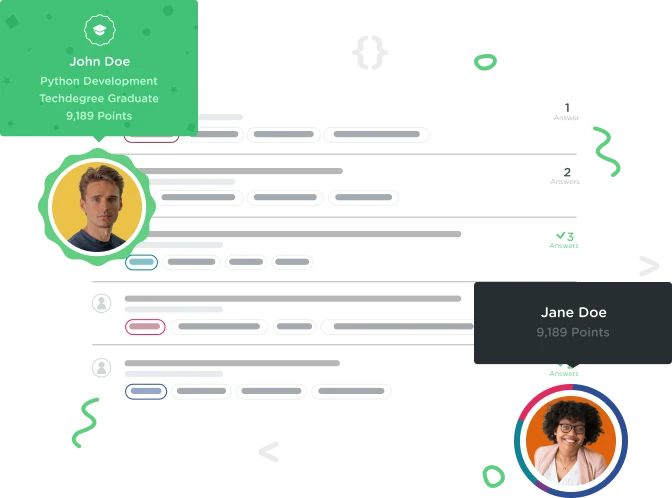

sj
9,651 Pointsloop index reset when using it in the slice() method
I want to slice the word "home" from the string someText with the slice() method using the loop index as the starting point, but i'm getting "find".
here is the code:
var someText = "find it find it find it find it home find it find it find it find it ";
for (i = 0; i < someText.length; i++) {
if (someText[i] == "h") {
var temp = someText.slice(someText[i],4);
document.write(temp);
}
}
2 Answers

Knut Ringheim Lunde
38,811 PointsHello, Joe!
The slice-method takes two parameters/argument that should be of type number. The first is required and corresponds to the start value, the second parameter is optional and specifies the end value (up to, but not including). With this in mind, let us review what you do when assigning the temp variable.
var temp = someText.slice(someText[i], 4);
Your first argument is someText[i], which would evalute to a letter. In your case it would evaluete to the letter 'h' because of the if-statement you have. Your second argument is 4, which is the correct type, but that argument specifies the end-index, not how many further "to the right" it should go. So you should rewrite that assignment to the following:
EDIT: I think the reason your code returned "find" is that javascript converts your first argument ("h") to 0. And then your slice-method returns the string from index 0 to index 3.
var temp = someText.slice(i, i + 4);
But I would also like to point out that it your goal is to fetch the word "home" from a string, you could do this more effienctly using other methods like indexOf and substring.
var someText = "find it find it find it find it home find it find it find it find it ";
// Making some variables holding the values needed
var searchWord = "home";
var startIndex = someText.indexOf(searchWord);
var endIndex = startIndex + searchWord.length;
var temp = someText.substring(startIndex, endIndex);
NB: You should be careful using the double equal operator (==), it can have some unexpected behaviours, and it is recommended using the tripe equal operator (===) instead. You can read more here:.
I apologise for my English, but I hope it can be to help :)

sj
9,651 PointsThank you so much Knut Ringheim Lunde, your answer was very helpful .