Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial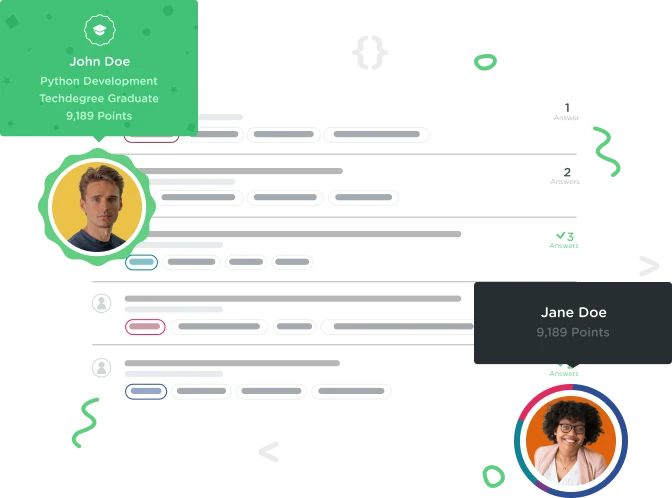
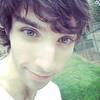
Alphonse Cuccurullo
2,513 Pointsloop issue
def get get = gets.chomp end
print " time to add to your array choose 3 items to store "
arr =[] puts arr
while arr.length != 3 puts arr.push(get()) if arr.length == 3 break end end
the goal here is to store three strings or numbers into the array by using the gets method an while loop. Any ideas what i did wrong?
2 Answers
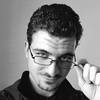
Houston Erekson
10,542 Points"gets" is already a method in ruby
answer = gets.chomp
and you should place everything you need inside your while loop. Try this
# Create an array
arr = []
# Print a line
print "Choose 3 items "
# Start the loop with a "<" and when the array has 3 items in it it will automatically stop
while arr.length < 3
# Print a line to notify input is needed
print "> "
# Get the answer from the user
answer = gets.chomp
# Add the answer to the end of the array
arr.push(answer)
end
# Print out the array so you can see what is in it.
print arr
Hope this helps
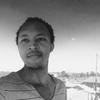
Dan Weru
47,649 Points# Note that "!=" stands for 'not equal to'
# You should have used "<=" for 'less or equal to'
# An if statement in your code was not entirely necessary
# Your code should have looked like:
arr = []
count = arr.length
while count <= 3
print "Enter Item"
item = gets.chomp
arr.push(item)
count+=1
end
#Alternatively,
arr = []
count = arr. length
while count <= 2
print "Enter Item"
item = gets.chomp
arr.push(item)
count+=1
end