Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial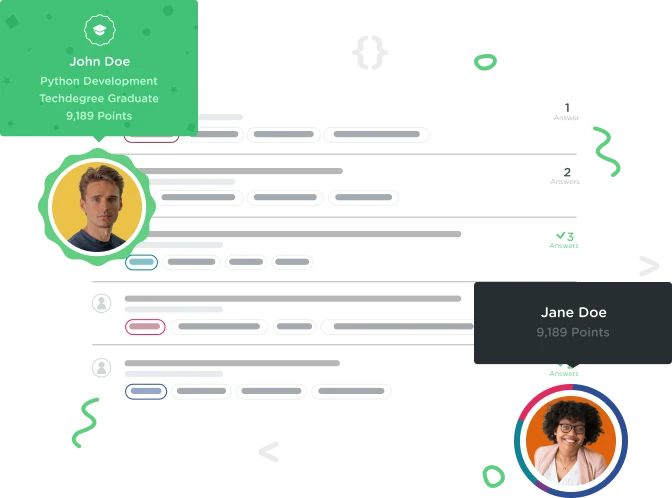

Oleksandra Smith
Front End Web Development Techdegree Student 9,361 PointsLoop question
The for loop in app.js cycles over list items and applies a color to each item using the values stored in the colors array. For example, it applies the first color in the array ( #C2272D) to the first list item, the second color (#F8931F) to the second list item, and so on.
Complete the code by setting the variable listItems to refer to an HTMLCollection. The collection should contain all list items in the <ul> element.
// Complete the challenge by writing JavaScript below
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
let list = document.querySelectorAll ('li');
for ( let i = 0; i < colors.length; i++ ) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript and the DOM</title>
</head>
<body>
<h1>My List</h1>
<ul>
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="app.js"></script>
</body>
</html>
1 Answer
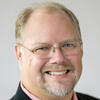
Jason Larson
8,361 PointsThere are 2 issues with your code. The first is that you changed let listItems;
to let list = ...
, so the second part of the script won't work because the variable name is wrong.
Having said that, your code would work in real-life, but the challenge specifically asks you to return an HTMLCollection, and querySelectorAll() returns a NodeList. You need to use getElementsByTagName()
:
let listItems = document.getElementsByTagName('li');