Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial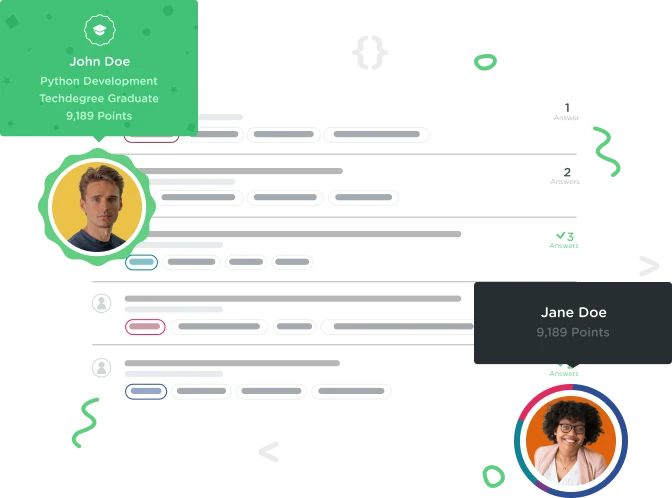

Jeremy Hester
1,021 Pointslooping different arrays from within one associative array, using one foreach loop?
Alright, the title probably sounds confusing so here's what I'm trying to do.
My array:
$lighting = array();
$lighting["Light 1"] = array(
"name" => "Light 1",
"paypal" => "XYZXYZ",
"display" => array("Red — $12.00","Blue — $12.00"),
"colors" => array("Red","Blue"),
);
My drop-down box code:
<select name="os0" id="os0">
<?php foreach($lights["colors"] as $color) { ?>
<option value="<?php echo $color; ?>"><?php echo $lighting["display"] ?></option>
<?php } ?>
</select>
My problem: I have to use the "colors" array within the "value" to send to paypal, otherwise my items will not be added to the cart. HOWEVER, I want to display a price next to each color choice in the drop-down. Like "Red - $12.00" and "Blue - $10.00" because different options might cost different amounts.
I could change each option in paypal, but that would be stupid to have the cost in the item name, and also very cumbersome. I've tried nesting two foreach loops within each other, and the output simply doesn't work. Is there another way I can do this?
3 Answers

Randy Hoyt
Treehouse Guest TeacherYou want to create each color as a nested array that contains the two options together, like this:
$lighting = array();
$lighting["Light 1"] = array(
"name" => "Light 1",
"paypal" => "XYZXYZ",
"colors" => array(
array("display" => "Red — $12.00", "value" => "Red"),
array("display" => "Blue — $12.00", "value" => "Blue")
)
);
When you are building the dropdown, you loop through each color like this:
<select name="os0" id="os0">
<?php foreach($lights["colors"] as $color) { ?>
<option value="<?php echo $color["value"]; ?>"><?php echo $color["display"]; ?></option>
<?php } ?>
Does that help?

Jeremy Hester
1,021 PointsUpdate to this, so you can kind of see what I'm trying to do...
When I change my dropbox code to look like this:
<select name="os0" id="os0">
<?php foreach($lights["colors"] as $color) { ?>
<option value="<?php echo $color; ?>"><?php foreach($lights["display"] as $displays) { echo $displays; } ?></option>
<?php } ?>
So here, I made another foreach loop inside of my original foreach loop. The second guy outputs the name of the light along with the the price to the dropdown. And it works, which is great, except this is the outcome: http://i.imgur.com/14zdVXw.jpg
I know there has to be some simple way to make this look like a regular dropdown, I can't think of it though.
This is how I want it to look: http://i.imgur.com/hsvybJl.jpg

Jeremy Hester
1,021 PointsRandy I could kiss you. That's perfect, thank you!
Randy Hoyt
Treehouse Guest TeacherRandy Hoyt
Treehouse Guest TeacherCome to think of it, it would probably be better for your two attributes to be "name" and "price", not "value" and "display". Maybe like this?
When you are building the dropdown, you can then specify how that data (color name and price) get displayed:
That keeps your code a little cleaner. You'll be keeping your display instructions ("show the color name followed by a dash followed by a dollar sign followed by the price") where it belongs.
Jeremy Hester
1,021 PointsJeremy Hester
1,021 PointsWhoops, I replied to the thread and not to you. That is perfect, thank you so much!
Randy Hoyt
Treehouse Guest TeacherRandy Hoyt
Treehouse Guest TeacherGlad to help!