Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial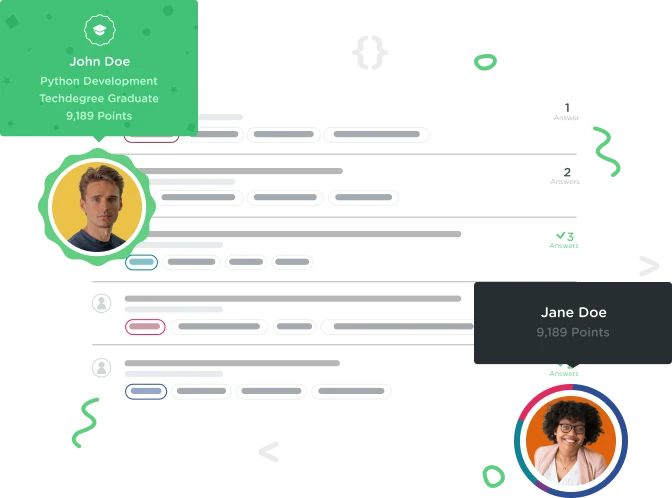

drstrangequark
8,273 PointsLooping if/else statement
I'm in a code challenge and it asks me to repeat the word "Yay!" as many times as was input by the user. I'm creating an if/else statement but can't figure out how to get it to loop if the total number of "Yays!" is less than the input. for example, I want something like:
if(total <= number) { Console.Write("Yay!"); total += 1; repeat; } else if(total == number) { break; }
Is there a command to do that? I can't find it on Google.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var total = 0.0;
bool keepGoing = true;
while (keepGoing)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Console.ReadLine();
var number = int.Parse(entry);
{
Console.Write("Yay!");
total += 1;
if(total <= number)
{
Console.Write("Yay!");
total += 1;
if(total <= number)
{
Console.Write("Yay!");
total += 1;
if(total <= number)
{
Console.Write("Yay!");
total += 1;
if(total == number)
{
break;
}
}
}
}
}
}
}
}
}
1 Answer
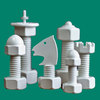
Steven Parker
231,269 PointsYou should use a loop to avoid repeating the same few code lines over and over. But the prompt and input and number conversion should not be inside the loop, they should each be done only one time.
Dominik Huber
4,631 PointsDominik Huber
4,631 PointsYes because right now you ask the user again and again after every new iteration through the loop.
So the way you can solve this is to get all the necessary data BEFORE you enter the while loop. In the while loop print "Yay!" for the number of times the user has put in. So you need some sort of loop count that you can set to 0 (an integer would be the best for this task (no need for a double)). Then you put the condition (when the while loop should stop) into the while loop:
(Also don't forget to increment (adding + 1) to the loop count variable. Otherwise you will end up in an infinite loop. No need for this keepGoing bool and you also don't need that many if statements.
You can do this :)