Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial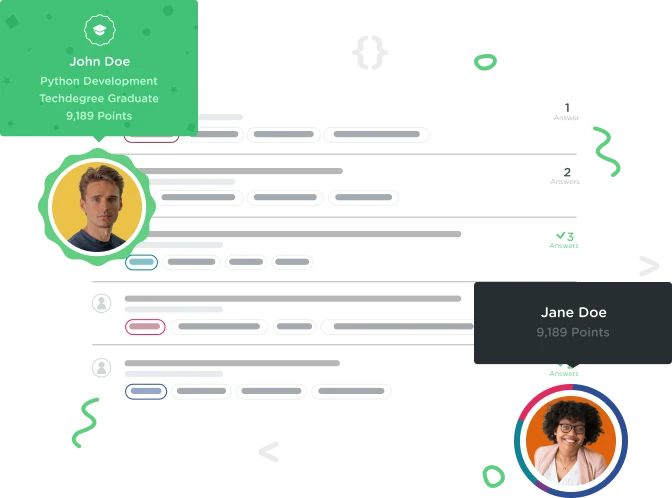

kirkbyo
15,791 PointsLooping threw multiple numbers in swift
I was wondering how I would go about looping threw numbers until the two numbers work. Ex: I want testNumOne and TestNumTwo to keep getting new numbers to see if it would work in the equation, then once a match is found it stops and logs those 2 numbers that work.
My Code
var ac = 20
var b = 9
var testNumOne = 5
var testNumTwo = 4
func isCommon (#numOne: Int, #numTwo: Int) -> Int? {
if numOne * numTwo == ac && numOne + numTwo == b {
return true
} else {
return nil
}
}
if let result = isCommon(numOne: testNumOne, numTwo: testNumTwo) {
println("Success - \(testNumOne) and \(testNumTwo) Work")
} else {
println("Failure - \(testNumOne) and \(testNumTwo) Does NOT Work")
}
Basically this code is supposed to find 2 numbers that can multiply to each other and those same two numbers can add to numbers specified.
Ex
I want "ac" equal to 20 and "b" equal to 9 So 10 x 2 equals 20 [10 x 2 = 20] But (10 + 2) does not equal 9 [10 + 2 = 12] The correct answer should be [5 x 4 = 20] [5 + 4 = 9] if that makes sense
So far the code mentioned above does that exactly but "testNumOne" and "testNumTwo" are static numbers and I want the numbers to find them self.
If you have any other questions or something is confusing about my explanation let me know :)
Thank you,
Ozzie
Any Ideas? Stone Preston Amit Bijlani Pasan Premaratne
1 Answer

Jeremy Hayden
1,740 PointsThe basic answer is that you can nest a Loop inside another Loop. This code will loop thru 0-100 for the second number for each of the first numbers. So your pair of numbers will be 0,0 0,1 0,2 0,3 ... then 1,0 1,1 1,2 ... then 3,0 3,1 3,2 ... ect.
for numOne in 0...100 {
for numTwo in 0...100 {
if let result = isCommon(numOne: numOne, numTwo: numTwo) {
println("Success - \(numOne) and \(numTwo) Work")
} else {
println("Failure - \(numOne) and \(numTwo) Does NOT Work")
}
}
}
But this will take 101 *101 or 10,201 loops to finish. We can shorten this drastically by using some logic. First, we don't need to go all the way to 100. If we are adding 2 numbers that will equal your variable b, then b is the upper limit that we need to check.
So the code can be modified to run shorter by changing the 100 to b.
for numOne in 0...b {
for numTwo in 0...b {
if let result = isCommon(numOne: numOne, numTwo: numTwo) {
println("Success - \(numOne) and \(numTwo) Work")
} else {
println("Failure - \(numOne) and \(numTwo) Does NOT Work")
}
}
}
This now cuts the looping count to about 100 loops for your example of b=9.
But with more logic, we can shorten it further. We do not really need the second loop because all we need to check for are number pairs that equal b. So if numOne is 1, then numTwo will be 8. If numOne is 2 then numTwo will be 7. We can calculate this with the following numTwo = b - numOne.
We can then modify the loop as follows.
var numTwo = 0
for numOne in 0...b {
numTwo = b-numOne
if let result = isCommon(numOne: numOne, numTwo: numTwo) {
println("Success - \(numOne) and \(numTwo) Work")
} else {
println("Failure - \(numOne) and \(numTwo) Does NOT Work")
}
}
This cut the loops down to 10 for your example.
Lastly, you can decide to stop the loop once your answer is found by utilizing a break statement.
var numTwo = 0
for numOne in 0...b {
numTwo = b-numOne
if let result = isCommon(numOne: numOne, numTwo: numTwo) {
println("Success - \(numOne) and \(numTwo) Work")
break
} else {
println("Failure - \(numOne) and \(numTwo) Does NOT Work")
}
}
This cuts your loop further in half to 5 for this example.
Your question was about looping, so I answered it with looping. But just FYI, I think the real solution your looking for is a quadratic equation formula.
kirkbyo
15,791 Pointskirkbyo
15,791 PointsThank you for your answer!