Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial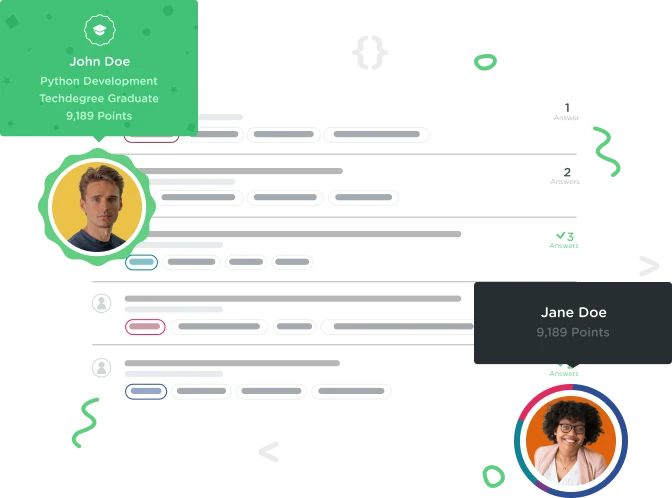

Daniel Silva
5,353 PointsLooping through an Object of Objects
I have an object that tell the user about the developer. I can loop through the main object and have the values printed out except for the hobbies object it prints "[object Object] ". Then when I try to do a nested loop I still get the above printed out but the hobbies print 12 times. Any suggestions.
var MeComplex = {
Name: 'Jon Doe',
Age: 27,
Married: true,
Classes: ['CSIS 2470', 'CSIS 1200', 'Math 1060', 'PHYS 1010'],
Hobbies: {
Hobby1: 'Camping. I love to relax and get away from the world sometimes.',
Hobby2: 'Canyoneering. Exploring a part of the world that not many people know exists.',
Hobby3: "Eating. I would die if I didn't do this."
}
};
var about = '';
var hobbies = '';
for (i in MeComplex) {
about += MeComplex[i] + '<br>';
if (MeComplex.Hobbies instanceof Object) {
for (k in MeComplex.Hobbies) {
hobbies += MeComplex.Hobbies[k] + '<br>';
}
}
}
document.getElementById('page').innerHTML = about + hobbies;
4 Answers
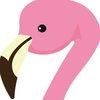
Dave StSomeWhere
19,870 PointsI see solutions while I was checking it out...
You're getting the object object because you are looping through all the index values (including the hobbies object).
Here's your code adjusted to work (I didn't fix the formatting):
var MeComplex = {
"Name":"Jon Doe",
"Age":27,
"Married": true,
"Classes":["CSIS 2470", "CSIS 1200", "Math 1060", "PHYS 1010"],
"Hobbies":{
"Hobby1":"Camping. I love to relax and get away from the world sometimes.",
"Hobby2":"Canyoneering. Exploring a part of the world that not many people know exists.",
"Hobby3":"Eating. I would die if I didn't do this."
}
}
var about = '';
var hobbies = '';
for (i in MeComplex) {
if (MeComplex[i] instanceof Object){
for (k in MeComplex[i]) {
hobbies += MeComplex[i][k] + '<br>';
}
}
else {
about += MeComplex[i] + '<br>';
}
}
document.getElementById("page").innerHTML = about + hobbies
Producing the following:
Jon Doe
27
true
CSIS 2470
CSIS 1200
Math 1060
PHYS 1010
Camping. I love to relax and get away from the world sometimes.
Canyoneering. Exploring a part of the world that not many people know exists.
Eating. I would die if I didn't do this.
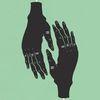
Ryann Brown
7,393 PointsThis is how I got it working. I checked to make sure that the trait wasn't Hobbies, and if it wasn't, I added it to about. Otherwise, it looped through the hobbies and added them to the hobbies variable. It was adding the hobbies 5 times, because it listed them once for each trait loop. Hope that helps!
let about = '';
let hobbies = '';
for (let trait in MeComplex) {
if (MeComplex[trait] !== MeComplex.Hobbies) {
about += MeComplex[trait] + '<br>';
} else {
for (let hobby in MeComplex.Hobbies) {
hobbies += MeComplex.Hobbies[hobby] + '<br>';
}
}
}
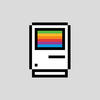
Hakim Rachidi
38,490 PointsHi Daniel,
To get the properties of an object is similar to getting the values from an array with the exception of that you need use Object.values(<object>) for the values and Object.keys(<object>) for the keys. The result is for both an array.
...
for (k in Object.values(MeComplex.Hobbies)) {
hobbies += k + '<br>';
}
...
Here is a reference
You can check if the current key is the Hobby like this
for (i in MeComplex) {
about += MeComplex[i] + '<br>';
if ( i === "Hobbies"){
for (k in MeComplex.Hobbies) {
hobbies += MeComplex.Hobbies[k] + '<br>';
}
}
}
Hope this helps;

Daniel Silva
5,353 PointsThank you Dave. I see now you need to check if there is another object first and add those before you just add the values of the original object the the about variable.