Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial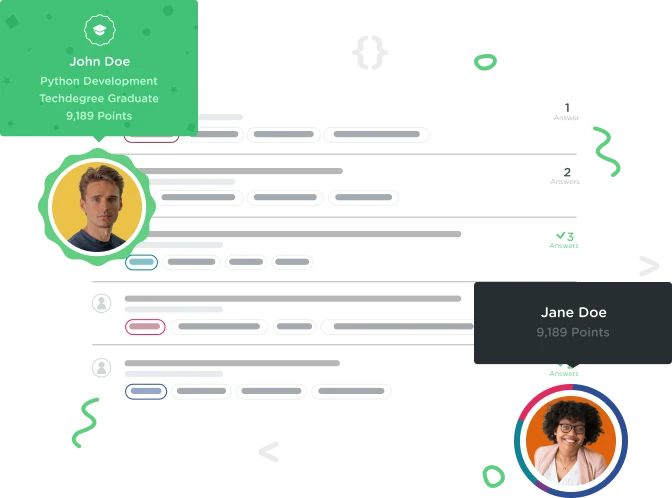

Grant Novey
10,352 PointsLooping through Objects in JavaScript
I'm playing around with creating objects in JavaScript. My goal with this exercise is to create an object that contains other objects. I want to loop through them so that I display the properties within the sister, mother, father, and pet objects.
I'm able to loop through the family object and I'm displaying that to the console. How do I get access to the other objects and their properties? I'm banging my head here.
var family = {
sister: {
firstName: "Sarah",
lastName: "Novey",
gender: "Female"
},
mother: {
firstName: "Mary",
lastName: "Novey",
gender: "Female"
},
father: {
firstName: "Bruce",
lastName: "Novey",
gender: "Male"
},
pet: {
firstName: "Dusty",
lastName: "Novey",
gender: "Male"
}
}
for(var person in family) {
console.log(person);
}
3 Answers
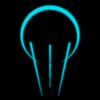
Jake Lundberg
13,965 PointsHere is the syntax that I use...
for(var mem in family) {
if (family.hasOwnProperty(mem)) {
var member = family[mem];
console.log(member.name);
console.log(member.age);
}
}

Grant Novey
10,352 PointsThank you! That worked. Now I just need to understand what's going on in the code :-)
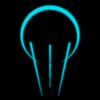
Jake Lundberg
13,965 Pointsill go line by line and explain:
for(var mem in family) {
This is cycling through the objects within the object.
if (family.hasOwnProperty(mem)) {
This is testing to see if the current object has properties of its own, and if they do...
var member = family[mem];
store the current object in the variable member
console.log(member.name);
console.log(member.age);
logging the name and age of the object to the console...
but as mentioned above, in this format, it would be better to use an array of objects...it would be easier to go through each of them that way. But in the future, if you have objects within objects, this is how you would go at accessing them...
Hope this helps!

Grant Novey
10,352 PointsThanks again! I've never heard of hasOwnProperty before. Good to know.