Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial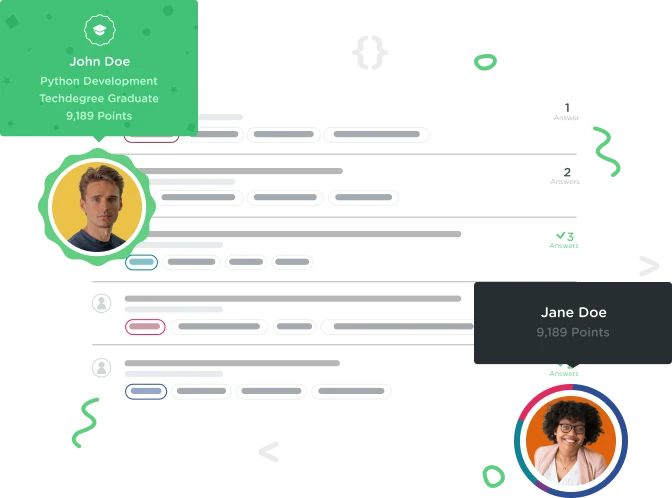

Christopher Kemp
3,446 Pointsloop.rb
Not really sure how to go about this, and I think some of that is because of how I am interpreting the instructions, but I could use some direction for this challenge.
def repeat(string, times)
fail "times must be 1 or more" if times < 1
counter = 0
loop do
print repeat(string)
counter + 1
if counter == times
break
end
end
end
1 Answer
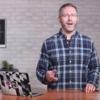
Jay McGavren
Treehouse TeacherA couple issues here.
First, you're actually making a call to the repeat
method from within the repeat method:
def repeat(string, times)
# ...
print repeat(string)
That's called "recursion", and it's probably not what you want to do. In this case, it's failing because repeat
is defined to take two parameters, but you're only passing one argument. But even if it worked, it would cause an infinite loop, as repeat
keeps calling itself. So don't call repeat
at all. Instead, you should just say: print string
.
Second issue is with this line:
counter + 1
That does add 1 to the value in counter, but then it doesn't do anything with it. For example, if I wrote:
counter = 0
counter + 1
...counter
would still be 0
. Instead, you want to write counter = counter + 1
, or better yet counter += 1
. That will actually update the counter
variable.
I made the above changes to your code, and it worked for me. Give it another shot!