Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial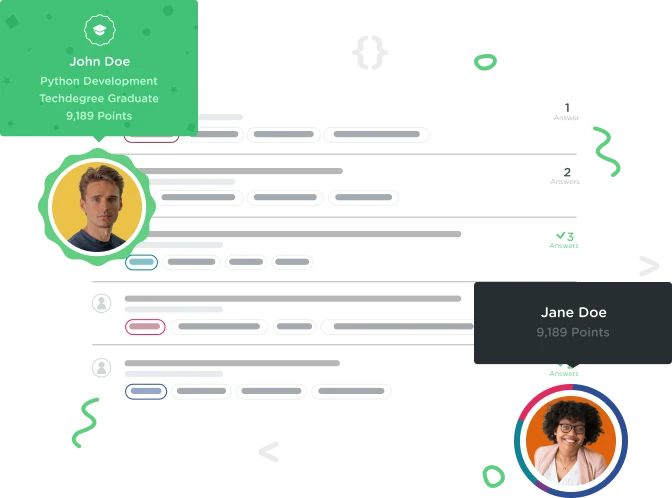
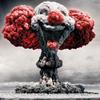
Gabriel Garcia
2,583 PointsLoops in Javascript
Hello So I was watching Jim's tutorials on loops in Javascript and i'm trying to make the extra credit but it seems like the output is not what I desire.
Here is the program instructions:
Extra Credit Fizz Buzz
Write a program that loops through the numbers 1 through 100. Each number should be printed to the console, using console.log(). However, if the number is a multiple of 3, don't print the number, instead print the word "fizz". If the number is a multiple of 5, print "buzz" instead of the number. If it is a multiple of 3 and a multiple of 5, print "fizzbuzz" instead of the number.
Hint. Use loops and if/else statments. In javascript the % is the modulo, or remainder operator. a % b evaluates to the remainder of a divided by b. 11 % 3 is equal to 2.
This is my code:
for (var counter = 0; counter <=100; counter++) {
if(counter%3==0){
console.log("fizz");
}
if(counter%5==0){
console.log("buzz");
}
if(counter%5 ==0 & counter%3==0){
console.log("fizzbuzz");
}
else{
console.log(counter);
}
}
Ive tried many things but i can't just make it work. If I just do it with numbers divisible by 3 without 5 and 5 and 3 it will work.
Any suggestions?
Thanks
Gabriel.
3 Answers

Gareth Borcherds
9,372 PointsYep, as Scott said, getting your if statements correct is a core part of programming. It's something that you hopefully get better at with practice. I would write the if statements like this for this task:
if(counter%5 ==0 && counter%3==0) {
console.log("fizzbuzz");
} else if(counter%3==0) {
console.log("fizz");
} else if(counter%5 ==0) {
console.log("buzz");
} else {
console.log(counter);
}
Basically, the reason I do the 5 and 3 is that you have to remember about if statements that the minute it reaches a true condition, it processes the true part and then stops processing. If you don't do it this way, it'll never get the 5 and 3 condition right.
This is a good example of how to think about the logic. I would recommend changing this if statement up and see what happens so you can learn more about them.

Scott Fletcher
236 PointsFirst, you'll want to use the javascript logical AND operator && (not the bitwise operator &).
Second, remember that an else
statement only gets associated with a single if
statement (and that an if
statement may contain 0 or more else if
s
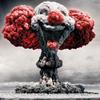
Gabriel Garcia
2,583 PointsThank you guys.
I understand now.