Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial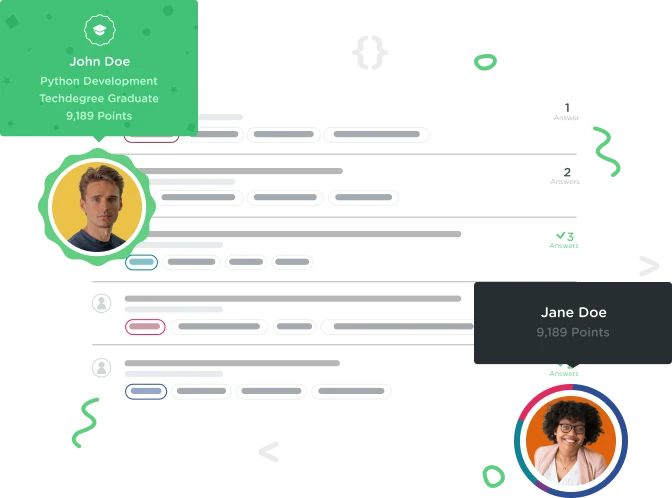

Henning Holm
4,163 PointsLoopy function(Break) and shopping list
I dont understand the question in challenge task "Break"? "...print each thing in items"? Isn't items just a placeholder for a value?
4 Answers

Alex _
17,107 Points"items" is whatever list is passed in to the "loopy" function. So, assume that items is a list. for example, it could be:
random_list = ["apples", "bananas", "onions", "milk", "ice cream"]
If that is the list, then calling "loopy" with this list:
loopy(random_list)
Would be expected to have this output (assuming the loopy function is defined elsewhere in the code):
apples
bananas
onions
milk
ice cream
So you want to write code that would loop through this list, or any other list which might be passed in, and print each value.

Mike Wagner
23,559 PointsIn this case, items is the iterable value that was passed into the function called loopy
like so
loopy(some_iterable_value)
the term items
is only a placeholder in the function definition, but once the function is called, it is actually representative of the argument passed into the function. You can sort of think of items
as a "temporary variable" that only exists when the function is actually running and reverts to None
when the function completes and returns, though that's a bit of an oversimplification, I think.
Say we have a variable courses
which is a list, like this:
courses = ["Python Basics", "Python Collections", "Functional Python"]
If we call loopy(courses)
then items
sort of becomes courses
and we are able to use a for-loop to iterate over items
and print out each member of the list.
Python Basics
Python Collections
Functional Python
We could even call loopy
like this
loopy([ "What is the answer to the Ultimate Question?", 42, "Look ma, no hands!"])
by defining what we pass into it when we call it, and it would still print off those items as before, as long as the argument passed to the function is iterable, you're in good shape. Since the Challenge wants that, it's safe to assume that they won't be passing any non-iterable items (unless they tell us to make sure on our own). It also makes our job easier, because all we need to do is define the function, not how we call it or what we call it with. All we need to do is define a for-loop and make sure we print a result, so it's all stuff we covered up to this point in the course :)

Henning Holm
4,163 PointsOkei, thanks, I think I get it :)
but there's another small thing
by writing:
for item in shopping_list: print(item)
Are you assigning a name(item) for the content of shopping_list?

Mike Wagner
23,559 PointsIf I'm understanding what you're asking, the answer depends on what you do with it. When you pass an argument into the function, the value in the function definition (in this case item
) sort of serves as an alias for that argument. By saying
for item in shopping_list:
print(item)
you aren't actually changing anything, you're only really using it as a reference that dies at the end of the function. You would actually have to assign that reference back into shopping_list
in order to change the members of the list. For example:
my_list = [2, 25, "hello"] # a test list
def loopy(items): # this items
for value in items: # becomes this items
print(value) # print the original value
value = 1 # set the value to 1
items = [] # set the entire items list to be empty
print(value) # print the value
print(items) # print the list
loopy(my_list)
#print(item) # adding these result in a NameError as
#print(items) #`item` and `items` are undefined outside the function
print(my_list) # print the original list to see unchanged
will output this:
2 # my_list[0]
1 # replaced w/ 1
25 # my_list[1]
1 # replaced w/ 1
hello # my_list[2]
1 # replaced w/ 1
[] # items = [] from above
[1, 25, 'hello'] # untouched my_list
You'll see two very important things. The first, and probably most important, is the fact that my_list
remains untouched even though we replaced value
and items
during the iteration process. The second, and slightly less apparent, thing is that even though we set the items
list to be empty in each iteration, once we reach the end of the loop, it goes back to being what it was. We can tell this because when we arrive at the print(value)
call in the second and third iteration, it actually gives us the value, rather than an IndexError
. This demonstrates the short lifespan of the variables (value
and items
) we defined within the loop.
There are several different ways to actually change the values in items
, but most of them are a bit more advanced than this topic allows for, and I'm far from an expert on them. I'll leave that up to someone else, in another thread to discuss, I just wanted to illustrate the "temporary" condition of value
or, in the original question item
. Hopefully that answered what you were asking and helped to make it a bit more clear. If I confused you more, you might check out this documentation about Python lists that Google threw together for developers. It's relatively easy to understand and fairly complete about the basics.

Henning Holm
4,163 PointsI understand, thank you for such comprehensive answers, Mike Wagner! :)

Mike Wagner
23,559 PointsNot a problem. Glad that I could help :)