Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial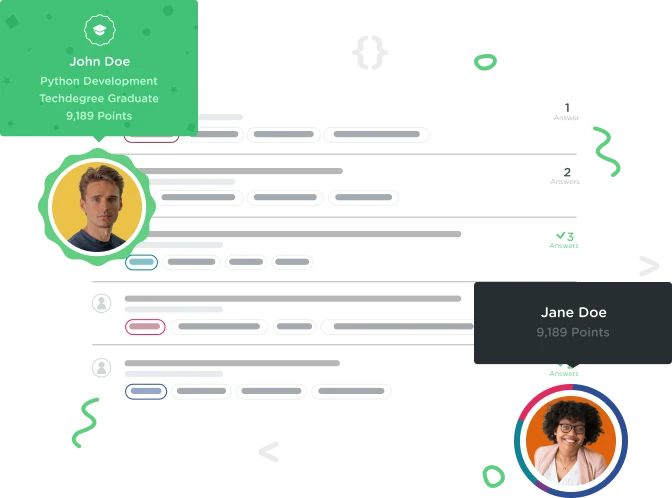
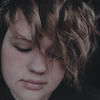
Ryan Maneo
4,342 PointsLost
I totally get what he's trying to do but I seem to have forgotten the syntax to do so, for the if instructions I would obviously use "if" and "else if" but I forgot how to pass in certain strings to return certain tasks... grrr!!!
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
/* Enter your code below
class Robot: Machine {
override func [What do I name it?](// What do I put for paramaters here?) {
if this then that?
else if this then that?
}
}
Super confused, help!
*/
2 Answers
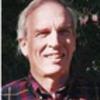
jcorum
71,830 PointsYou need to override the move method, which in Machine just prints. You need a switch statement to switch on the direction being passed in as a parameter to the method. As the instructions say, the directions are either Up, Down, Left or Right. For each direction you need to increment or decrement the x or y value of location:
class Robot: Machine {
override func move (direction: String) {
switch direction {
case "Up":
self.location.y++
case "Down":
self.location.y--
case "Left":
self.location.x--
case "Right":
self.location.x++
default: break
}
}
}
Note, you must have a default for the switch. Although location.y += 1 is the same as location.y++, the editor won't take the former.
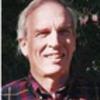
jcorum
71,830 PointsOne thing I forgot to mention is that if you don't remove the apostrophe from this line:
print("Do nothing! I'm a machine!")
your code will not be colored correctly in the editor. It will look like a big comment (all orange). But the editor will still accept it.
I also didn't answer two of the questions in your code: What do I name it? What do I put for parameters?
When you override you are either replacing or revising an inherited function. You must name the overriding function exactly the same as the function you are overriding, and it must have exactly the same method signature (number and type of parameters).
Not sure why you are getting the error you report. If you copy my code above, and paste it directly under this comment: // Enter your code below it should work fine. I just tried it again, and got no error message.
The error you report is very puzzling, as this code doesn't call any function. Are you trying to call the move() function somewhere?
Hope this helps.
Ryan Maneo
4,342 PointsRyan Maneo
4,342 PointsStill nope... error is: swift_lint.swift:22:45: error: function produces expected type 'String'; did you mean to call it with '()'? print("Do nothing! I'm a machine!") { ^