Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial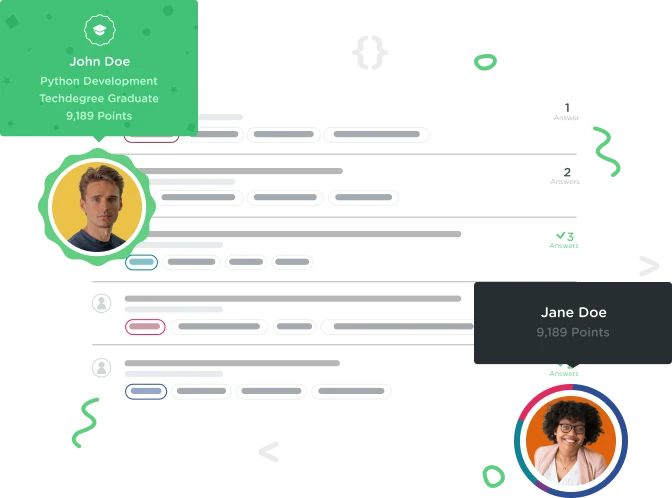
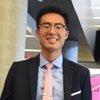
Paul Je
4,435 PointsLost!
Not sure where I've went wrong. Any help would really be appreciated!
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(firstPost) iOSDevelopment by Apple. Filed under swift"
}
}
let firstPost = Post(title: "Catching Fire", author: "Susane Collins", tag: Tag(name: "#hashtagawesomebestsellingbook"))
let postDescription: String = firstPost
3 Answers

Greg Kaleka
39,021 PointsHi Paul,
There are two lines with problems:
Line 1 is the return statement in your description()
method. It's a method inside the Post class, so it doesn't know anything about firstPost. More broadly, you want this description to describe any instance of Post. So we have to avoid hard-coding in things like "Apple" and "swift". Instead, the return statement should include whatever the post's name, author, and tag name are. To do this, you want to interpolate those properties like this:
"\(title) by \(author). Filed under \(tag.name)"
Hopefully that makes sense.
Line 2 is the last one. You don't want to set postDescription to equal the entire Post object firstPost
. You only want the description stored in that variable. You'll use the description()
method to get it. I'll leave the implementation to you.
Let me know if you're still stuck!
Happy coding
-Greg
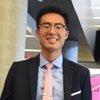
Paul Je
4,435 Pointsstruct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(name)"
}
}
let firstPost = Post(title: "Catching Fire", author: "Susane Collins", tag: Tag(name: "#hashtagawesomebestsellingbook"))
let postDescription = description() -> String
I'm not sure how to implement exactly (sorry for being a noob), is there an answer I could see? I got your first part loud and clear though, and now thing start to make sense!

Greg Kaleka
39,021 PointsHey Paul,
OK, so description()
is an instance method in the Post class. That means it's called on an instance of Post, like, for example, your constant, firstPost
.
The way you've written your last line of code tells me you need a refresher on object oriented Swift. I recommend at the very least reviewing this video on Classes (skip ahead to 6:40 if you just want to see a review of methods), and perhaps watching the whole course if you haven't already. You could also do some googling on "instance methods Swift" to get some more exposure.
Good luck! I hope you don't mind that I'm making you work for this a bit . I've found that usually, if I'm having trouble with a challenge, it's because I need to review something.
Happy coding
-Greg
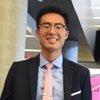
Paul Je
4,435 PointsThanks Greg! I've got it and that really helped a ton.