Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial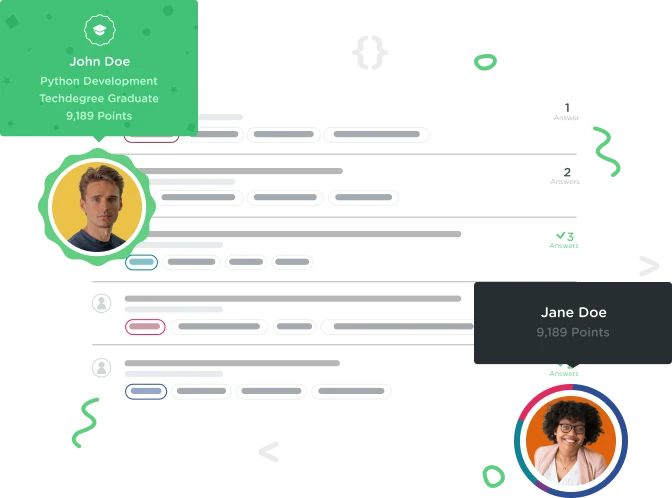

Nazeim Brame
6,544 PointsLost
This entire section has been confusing me. I'm struggling to grasp the concept.
var inputValue = document.getElementById('linkName').value;
var inputValue= linkName.textContent
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<label>Link Name:</label>
<input type="text" id="linkName">
<a id="link" href="https://teamtreehouse.com"></a>
</div>
<script src="app.js"></script>
</body>
</html>
3 Answers
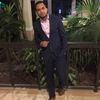
Xavier Ritch
11,099 PointsHope you got this one figured by now but if not, the challenge task is asking you to set the textContent of the <a> tag equal to the value of the inputValue variable. So first we would select the <a> tag with getElementById and then call textContent on it, then store that in our variable So it should look something like this:
var inputValue = document.getElementById('linkName').value;
document.getElementById('link').textContent = inputValue;
hope this helps!
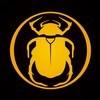
rydavim
18,813 PointsOkay, so assuming you're working on Task 2 you'll need to be setting the text value, rather than creating a new variable. Here's some pseudo-code to get you started.
// use the same document.getElementById that you've used previously to grab the element 'link'
// you'll then want to chain it into a method for text content (textContent)
// and set that equal to the variable you created in the first task
If you're having trouble with the syntax, try taking a look at the w3 page for textContent.
Hopefully that helps get you on the right track, but please let me know if you still have trouble and we can work through a solution together. Happy coding!

Nazeim Brame
6,544 Pointsvar inputValue = document.getElementById('link').textContent;
How do I set it equal to the inputValue variable?
I tried
let input = inputValue;
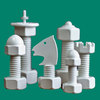
Steven Parker
229,786 PointsTask 2 says, 'Next, set the text content of the <a> element with the ID link to the value stored in inputValue."
So the left side of the assignment will select the element with the ID of "link", and access the text content attribute, and the right side of the assignment will be the value you got in task 1 ("inputValue").
And you won't need another "let" or "var" since no new variables are being created.

Nazeim Brame
6,544 Pointsvar inputValue = document.getElementById('link').textContent;
How do I assign the value from task 1 onto the right side??? It's confusing saying it like that because I thought there could only be 1 '=' per line of code.
Would it be
var inputValue = document.getElementById('link').textContent = ("inputValue"); ???
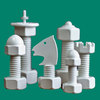
Steven Parker
229,786 PointsCloser, but you don't re-create the variable so you'll have only one assignment operator:
document.getElementById('link').textContent = inputValue;