Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial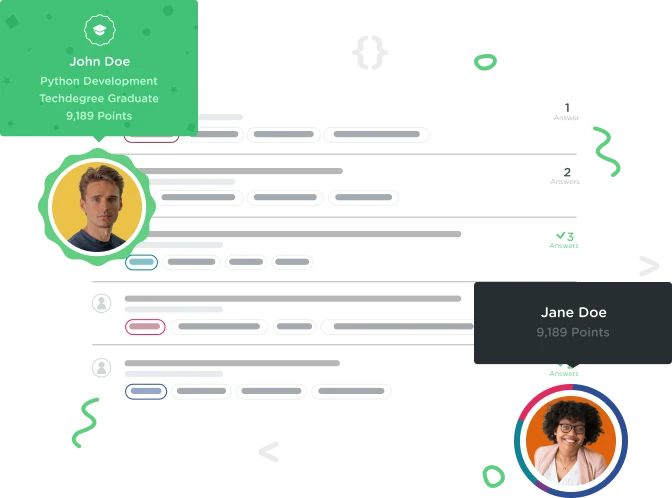
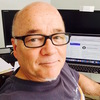
Bob Amand
2,639 PointsLost on counting Keys.
Clearly I am missing the point of how to count the keys in the dict and the list. I have reviewed lists, videos and tried many many approaches. Now I am confusing self. I suspect there is something wrong with how I am cycling through the list. A clue would be welcome.
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
def members(my_dict, my_list):
count = 0
for key in my_dict:
if key == my_list:
count += 1
return count
4 Answers
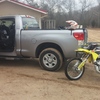
Ryan Ruscett
23,309 PointsHola,
So yeah, this can be a bit tricky. Especially between Python 2.7 and Python 3.x. Python 2.7 has iterkeys() and has_keys() which are no longer available in 3.4. That being said, there are a billion ways to do this one thing lol. Let's break down only 1 example.
I have a dictionary that is Key/Value. I need to find a way to iterate over just the keys in the dict. To the python interpreter I go. Or Treehouse workspaces.
1. I can use dir(dict) in workspace or python iterpreter.
dir(dict)
['__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'clear', 'copy', 'fromkeys', 'get', 'items', 'keys', 'pop', 'popitem', 'setdefault', 'update', 'values']
Ok, now what in here looks good. Let me try "keys" What does that do? I can use help for this. Back to the interpreter.
>>> help(dict.keys)
Help on method_descriptor:
keys(...)
D.keys() -> a set-like object providing a view on D's keys
Ok, well a set like object. That is ok, since sets do not contain duplicates and are immutable. Well so are dictionary keys. This sounds like what I want. Now I need it in a loop. Hmmm I can do a for loop, while loop well. a for loop makes more sense to me. I don't need to do something while something else is occuring. I just need to do this one thing. We will leave it at that and I will spare you on iterator vs iterable and generators and why for is blah blah don't want to lose you yet lol.
for keys in my_dict.keys():
Ok, so now I will iterate over the dictionary and set the dictionary keys to the variable key. As I move through the dictionary key will change with each iteration until the end of the loop or "stopiteration" is raised. That's handled behind the scenes by python so we don't care about that.
Now, I need to check if the key exists inside the list. I can use "in" or membership or whatever you want to call it.
if key in my_list:
Awesome! So now I just need to do something if it is. How about I start a counter, and set it to 0. I want it outside the loop but inside the function. This has to do with the scope which we wont get into here.
If true add 1 if false do nothing or pass which is a python keyword to do nothing. Then return the count.
OH can't forget to put it all in a function.
def members(my_dict, my_list):
count = 0
for keys in my_dict.keys():
if key in my_list:
count += 1
else:
pass
return count
Wallah! We are finished.
I hope this helps, if not let me know and I can try to explain it better lol.
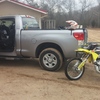
Ryan Ruscett
23,309 PointsHey Andrea,
Sure no problem. In a dictionary like the one below from the python docs.
tel = {'jack': 4098, 'sape': 4139}
The dictionary above is comprised key/value pairs. In other words, there are two things that make up a dictionary.
- Key's (jack, sape)
- Values (4098, 4139)
The above example has two keys. jack and sape. It also has two values 4098, 4139. A key is a reference to a variable or value inside of a dictionary. You can think of it like assigning a variable.
number = 1
Number is a reference to 1. Well, in a dictionary the key is a reference to the value.
This is good for property files. Take this for example.
I have a property file called DatabaseProperties
hostname = localhost port = 5555
The properties file has two key value pairs. The keys are hostname and port. While the values are localhost and 5555. I could stick the two key/value pairs inside a dictionary. Than anywhere in my program that I needed to call the hostname. I could retrieve the value of the key.
DBConnection = {"hostname": localhost, "port": 5555}
DBConnection['hostname']
localhost
DBConnection['port']
5555
See, I can call a value inside my dictionary based on the key. This is why dictionaries can't have duplicate keys. Or else it wouldn't know what value to get. The key is a reference to a value and what it returns is the value associated with the key. Just like number returns 1
Keys have several rules associated with them. For instance you can't have duplicate keys as mentioned above. There are ways to combine dictionary values when you are trying to add a key/value pair to a dictionary where the key already exists. But we wont get into that here.
Does this help you better understand what a key is? Please let me know if not and I can try and explain it another way.
Thanks!
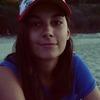
Andrea Campos
4,743 PointsThank you SO MUCH Ryan! This has been extremely helpful. I understood it perfectly :) Have a great day! A.
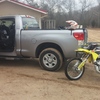
Ryan Ruscett
23,309 PointsNo problem. "in" Is membership. There are two kinds really. "In" and "not in". Each one returns a True and or False. If you run into any questions along the way or with your research. Just let us know! Thanks!
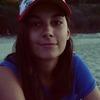
Andrea Campos
4,743 PointsHello Ryan! I found your answer really helpful, but I still can't understand the meaning of a 'key'. Can you explain it to me, please? Thank you so much! A.
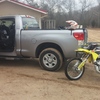
Ryan Ruscett
23,309 PointsI tried answering your question, but it moved it up towards the top of the thread. let me know if it works for you. Thanks!
Bob Amand
2,639 PointsBob Amand
2,639 PointsThank you for the comprehensive answer. I not only followed you perfectly, I hope to apply your logical approach to other problems. Also, I always seem to want to use an equal sign instead of "in" within the "if" statement. I will need to research the distinction on my own. Thanks. Bob