Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial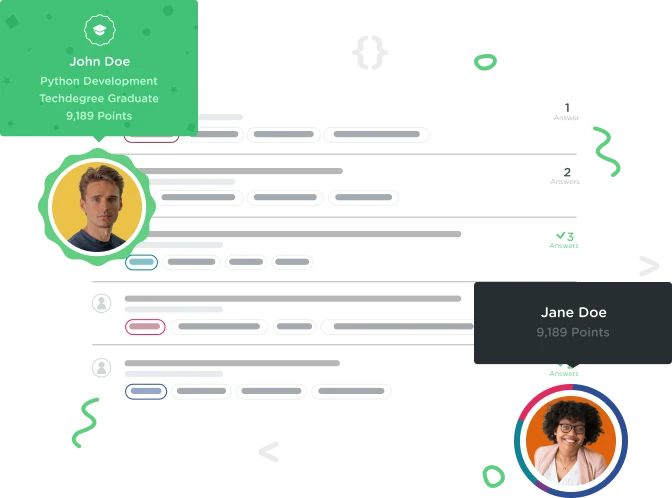
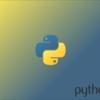
Kevin Faust
15,353 PointsLost when we started coding Prompter.java....
Hi everyone
Ive got a few questions
(1) I dont really get this part:
public Prompter(Game game) {
mGame = game;
}
so in the main file we create a prompter object with "game" as a constructor parameter. so this "game" is the object right? and then in our prompter file, i can visualize as "game" going into (Game "game") replacing the parameter value. And because we have our "Game" class there, that signifies its a object? so we always have to put our object class there? and thats why our instance variable also looks like this: Game mGame..So its safe to assume in order to preserve it as an object, we always put the class name. Sorry ive never seen putting objects into parantheses before and its confusing...
(2) What even are we doing here:
Console console = System.console();
Craig said some stuff about system packages and thats why we had to put the import statement. Is this something that we must know now or is this just something to make our program work for now and we will go over this later on?
(3) Also why cant we have just written this:
char guess= console.readLine("Enter a letter: ");
instead of this:
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
Is the first way wrong? Do we have to store our response as a string first and then convert it a char after that?
(4) and then our last line i just want to confirm
So we basically call the applyGuess method from the mGame variable which contains the game object and we insert our character guess into it. And this will run the method on the other file page and return a boolean value as well as adding the guess to "mHits" or "mMisses"
(5). Also why couldnt we just keep everything in the Game.java file page? meaning that we put all of this inside of game.java:
import java.io.Console;
public boolean promptForGuess() {
Console console = System.console();
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
I was trying to make this work because i was so confused with the above questions but i failed. but are we just dividing everything up for easier access and its more easier to read code? because that would make sense.
Any Help would be greatly appreciated. Thanks
1 Answer

Daniel Hartin
18,106 PointsHi Kevin
Wow that took some time to read.... :)
I'll try and answer as much as possible.
Passing in an object as a parameter - I assume you have created methods before that pass in regular variable such as string and int etc etc. There is no real difference with passing in an object only that we can reference all of the methods and parameters of the object from inside the new method. This si especially useful when you want to pass in and export several values from one method you can simply create a convenience class to define all these variable in and then edit them and send back the edited object (hopefully this makes sense). What I think is happening here is the new class wishes to exploit this ability of 'grouping' a load of variable together in a 'convenience' class and is therefore asking for it as a parameter, you are then setting this as a member variable of the new class (defined by the m prefix) so you can reuse all of its methods and variable through the new class (clear as mud... don't worry it makes sense after a few night swearing at the screen)
The console variable is simply for convenience you could have used the fully qualified reference System.console.readline() but to avoid typing the full reference each time you can assign the returned Console class in a variable to reuse later, there are a number of instances in Java where this chaining together of objects makes for shorter code writing. Basically each object returns an object (like the 1st method above) from the method call so you can keep calling methods from the returned objects.
console.readLine() returns a string and as a result will not fit into char as this is a single character, as you have shown in your example it is split into 2 separate line but..... as mentioned twice now you can take advantage of the chaining if you wish and use console.readLine().charAt(0); the console.readLine returns a string which you don't have to assign to a variable you can simply call all the methods off of the returned String object which is returned by readLine().
Lastly splitting things up especially at the beginning seems unnecessarily clunky and over complicated I'd agree but if this were a bigger project and you returned to it several months later it makes it much easier (with well commented and well written code) to identify any potential bugs you might have encountered or to make changes. The other bonus is by separating out code you make each method unreliant on others and therefore any error made by one method shouldn't have any impact on others i.e. if a method takes in a string as a parameter and returns an int there is no way it will try to work if you pass in an int making it easier to identify the problem.
Wow, I think that's about as clear as mud! hopefully you can decipher something out of all that text
Let me know if you want me to go over anything
Thanks Daniel
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsHey Daniel,
your response was helpful. I get how we are passing an object but it just looks foreign because we are no longer using String, int, boolean but rather a class name. Im sure ill get over this with time though. I also wanted to check one more thing. If we passed in (String game) instead of (Game game), this would pass in a string text to mGame rather than the object game. So when dealing with objects like in this file, we should always have the class name in the parameter and in the instance variable if it is a object.
My attempt at chaining but im not sure if i did it right:
Daniel Hartin
18,106 PointsDaniel Hartin
18,106 PointsHi Kevin
if you defined your method parameter as String game the parameter type would be a string which would cause an error when you tried to pass in a Game object, this is highlighted in most modern IDEs with a red underline which helps to prevent you using the wrong data types and is the entire reason for separating code. An example of qhat I was explaining yesterday is below.
The HolderClass can be created simply to hold 3 variables, now I could pass these variable in separately each time but it starts to look messy with so many parameters being passed in so my preference is to create HolderClasses at times to neaten up the code this way you can pass in an entire object and then reference the variables assigned to the object as per below.
If you wanted to pass in a string instead you would have to pass in the string representation of the class by calling the toString() method like game.toString() but yes in principle you are correct. Just to be clear the String data type you have been using is actually a class (hence the uppercase first character) which is why you can use methods such as .equals() and .charAt() methods directly off of the string variable, defining the data type to be one of your own classes is exactly the same principle.
In case you haven't spotted it you were nearly there only you missed the parenthesis of the console method.
char guess = System.console().readLine("Enter a letter: ").charAt(0);
Hope this helps Daniel
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsThanks a ton Daniel. Cheers