Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial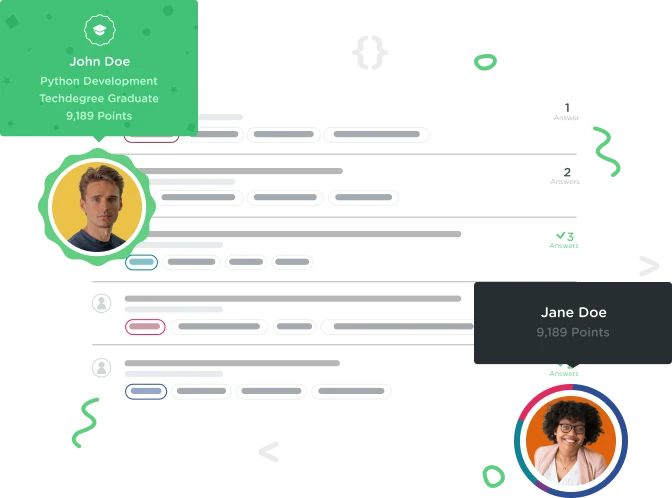

Raul Cisneros
7,319 PointsLots of DRY code but it works and has the fix for incorrect name entry and blank entry.
var student = [
{name: "Susan", track: "Web Design", achievements: 2, points: 25},
{name: "Melissa", track: "Full Stack Dev", achievements: 3, points: 50},
{name: "Sarah", track: "Front End Dev", achievements: 4, points: 75},
{name: "Jennifer", track: "IOS",achievements: 5, points: 100},
{name: "Alexia", track: "Python", achievements: 6, points: 125}
];
function print(x) {
document.getElementById('output').innerHTML = x;
}
while (true) {
var userInput = prompt("Enter student name. Type 'quit' to exit");
userInput = userInput.toLowerCase();
if (userInput === "quit") {
break;
} else if (userInput === "susan") {
susan();
} else if (userInput === "melissa") {
melissa();
} else if (userInput === "jennifer") {
jennifer();
} else if (userInput === "sarah") {
sarah();
} else if (userInput === "alexia") {
alexia();
} else if (userInput === "") {
alert("Please try again");
} else if (userInput !== "susan", "alexia", "melissa", "jennifer", "sarah", "quit") {
alert("No records by that name.")
}
}
function susan() {
for (var i = 0; i = 5; i++) {
var message = '';
message = message + '<h3>' + 'Student name: ' + student[0].name + '</h3>';
message = message + '<p>' + 'Track: ' + student[0].track + '</p>';
message = message + '<p>' + 'Acheivments: ' + student[0].achievements + '</p>';
message = message + '<p>' + 'Points: ' + student[0].points + '</p>';
print(message);
break;
}
}
function melissa() {
for (var i = 0; i = 5; i++) {
var message = '';
message = message + '<h3>' + 'Student name: ' + student[1].name + '</h3>';
message = message + '<p>' + 'Track: ' + student[1].track + '</p>';
message = message + '<p>' + 'Acheivments: ' + student[1].achievements + '</p>';
message = message + '<p>' + 'Points: ' + student[1].points + '</p>';
print(message);
break;
}
}
function sarah() {
for (var i = 0; i = 5; i++) {
var message = '';
message = message + '<h3>' + 'Student name: ' + student[2].name + '</h3>';
message = message + '<p>' + 'Track: ' + student[2].track + '</p>';
message = message + '<p>' + 'Acheivments: ' + student[2].achievements + '</p>';
message = message + '<p>' + 'Points: ' + student[2].points + '</p>';
print(message);
break;
}
}
function jennifer() {
for (var i = 0; i = 5; i++) {
var message = '';
message = message + '<h3>' + 'Student name: ' + student[3].name + '</h3>';
message = message + '<p>' + 'Track: ' + student[3].track + '</p>';
message = message + '<p>' + 'Acheivments: ' + student[3].achievements + '</p>';
message = message + '<p>' + 'Points: ' + student[3].points + '</p>';
print(message);
break;
}
}
function alexia() {
for (var i = 0; i = 5; i++) {
var message = '';
message = message + '<h3>' + 'Student name: ' + student[4].name + '</h3>';
message = message + '<p>' + 'Track: ' + student[4].track + '</p>';
message = message + '<p>' + 'Acheivments: ' + student[4].achievements + '</p>';
message = message + '<p>' + 'Points: ' + student[4].points + '</p>';
print(message);
break;
}
}
1 Answer
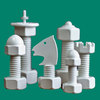
Steven Parker
229,732 PointsYou could make this code a bit more "DRY" and about half the size by combining the similar functions a single one that takes a parameter (and it also does not need a loop).
For example:
function showStudent(number) {
var message = '<h3>Student name: ' + student[number].name + '</h3>';
message += '<p>Track: ' + student[number].track + '</p>';
message += '<p>Acheivments: ' + student[number].achievements + '</p>';
message += '<p>Points: ' + student[number].points + '</p>';
print(message);
}
Then, instead of calling the different named functions, call the single one and pass the student number:
} else if (userInput === "susan") {
showStudent(0);
} else if (userInput === "melissa") {
showStudent(1);
// etc...
Raul Cisneros
7,319 PointsRaul Cisneros
7,319 PointsAfter watching the solution video it really makes me feel like I don't know anything. I know my solution is not the best way to code, but it does work and with time I am hoping that I can code something similar to the instructors solution. I figure that if I cant figure it out in a couple days, its time to move on.