Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial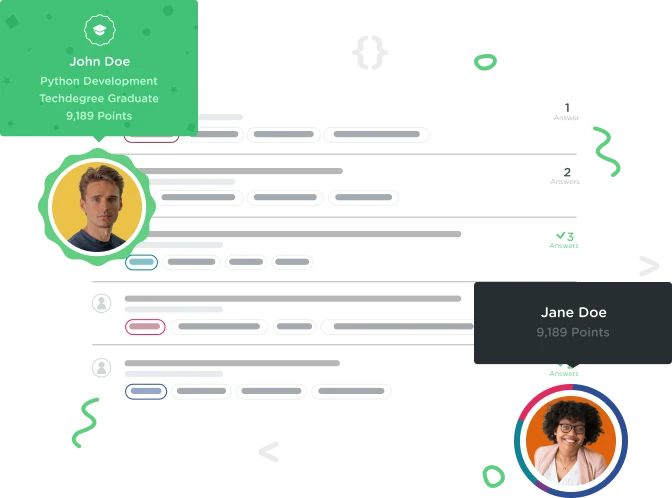

Liam George
12,004 PointsLots of errors with C++; how do I fix them?
So I know C++ isn't offered on Treehouse, but I'm really hoping someone can help me. I have an assignment where I need to make a programme that needs to display the two best phone plans based on the data provided. Here is the code with errors:
using namespace std;
Bill* createBill(const Customer& billHolder, const PhonePlan& billPlan, const int& callCount, const PhoneCall history[0]);
PhoneCall history[0];
int getTotalHours() { // Method: Hours = Seconds / 3600 int getTotalHours(const Bill& bill); return history[0].getCallSeconds() / 3600; }
int getTotalMinutes() { // Method: Minutes = Seconds - (Hours * 3600) / 60 int getTotalMinutes(const Bill& bill); return history[0].getCallSeconds - (getTotalHours()* 3600) / 60; }
int getTotalSeconds() { // Method: Seconds = Seconds - (Hours * 3600) - (Minutes * 60) int getTotalSeconds(const Bill& bill); return history[0].getCallSeconds - (getTotalHours() * 3600) - (getTotalMinutes() * 60); }
double getCalledCost() { // Method: Called Cost = Plan Block * Plan Rate double getCalledCost(const Bill& bill); return history[0].getPlanBlock * PhonePlan.getPlanRate; }
double getTotalCost() { // Method: Total Cost = Called Cost - Plan Alowance double getTotalCost(const Bill& bill); if (PhonePlan.planAllowance > 0) { return getCalledCost - PhonePlan.phoneAllowance; } else return 0; }
void getTwoBest() { for (int i = 0; i < getTotalCost() - PhonePlan.monthlyFee; ++i) { if (getTotalCost() + PhonePlan.monthlyFee < getTotalCost() + PhonePlan.monthlyFee) { cheapestPlan() = getTotalCost() + PhonePlan.monthlyFee } else (getTotalCost() + PhonePlan.monthlyFee < getTotalCost() + PhonePlan.monthlyFee) { secondCheapestPlan() = getTotalCost() + PhonePlan.monthlyFee } } }
Basically the the errors are: 1) No matching constructor for initialisation of "PhoneCall [0]" 2) "PhonePlan" does not refer to a value 3) Cannot use dot operator on type, and 4) Variable declaration in condition must have initialiser.
Thanks!
1 Answer

james white
78,399 PointsHi Liam,
More useful than posting your C++ code is posting the information
about what IDE (Integrated Development Environment) you are using.
For instance I use the Microsoft Visual Studio .Net IDE for my C/C++ debugging.
If has a whole variety of debugging tools including Intellisense, viewing the Call Stack and even WCT (wait chain traversal to prevent multi-threaded deadlocking) .
You could be using any IDE to debug your C++ code:
Eclipse, NetBeans, CodeLite, Borland, Dev C++, QT Creator or any number of C++ IDES.
Important Note:
Each IDE has it's own debugging (and error correcting) tools.
The various IDE debuggers even usually have settings for the level of verboseness,
whether bad code generates a debugging level of warning or error,
and the VS.Net even links all errors found into the corresponding MSDN documentation.
Better than begging/using fellow Treehouse members to fix/debug your errors
is taking the opportunity to learn to use your IDE to help you figure out
why the errors are being generated so you don't continue to write code
that generates the same errors in the future (and makes you a more productive coder).
I could never give you all the information you need
to debug errors in C/C++ code in a single TeamTreehouse forum thread.
That really only comes with experience.
However, it is such a large topic that even whole books are written about it:
http://www.amazon.com/Debugging-Troubleshooting-Programmers-Chris-Pappas/dp/0072125195
http://www.amazon.com/Practical-Debugging-Ann-R-Ford/dp/0130653942
http://www.amazon.com/Inside-Windows-Debugging-Developer-Reference/dp/0735662789