Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial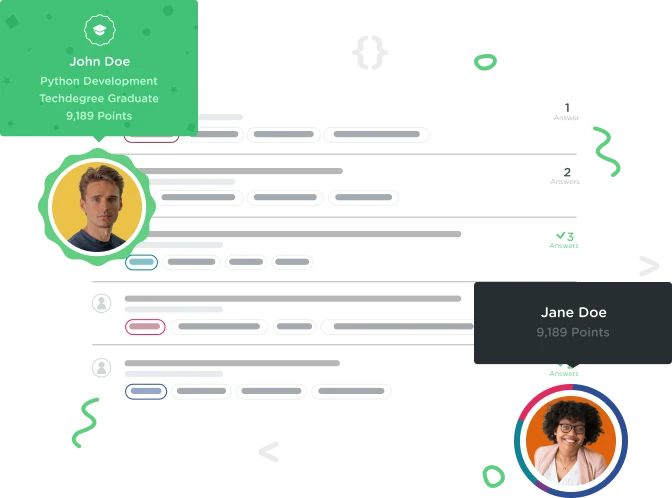

Victor Katsande
3,094 PointsMADE A COUPLE OF CORRECTIONS. STILL TELLING ME TASK ONE IS NO LONGER PASSING.
HERE IS THE ORIGINAL QUESTION
Alright, last step but it's a big one.
Make a while loop that runs until start is falsey.
Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99.
If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number.
Finally, decrement start by 1.
I know it's a lot, but I know you can do it!
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start > 0:
num = random.randint(1, 99)
if even_odd(num) == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -+ = 1
1 Answer
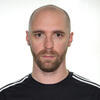
Robert Ionut Muraru
Full Stack JavaScript Techdegree Graduate 22,194 PointsThe below syntax is not correct:
start -+ = 1
You want to use:
start -= 1
You need no spaces between minus and equal and the plus sign should not be there. You will then have to check the logic in the if / else statements, as it is printing the opposite of what is required:
if even_odd(num) == 0:
print("{} is even".format(num))
- the above is the same as saying to python: if even_odd() is False...print out that the number is even. Remember that 0 is falsey. The even_odd() function checks if the number is even and returns True if it is even.
Malvin Mahati
7,555 PointsMalvin Mahati
7,555 Pointsthis should work
def even_odd(num): # If % 2 is 0, the number is even. # Since 0 is falsey, we have to invert it with not. return not num % 2
def start(): start = 5
while start: num = random.randint(1, 99) if even_odd(num): print('{} is even'.format(num)) else: print('{} is odd'.format(num)) start -=1