Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial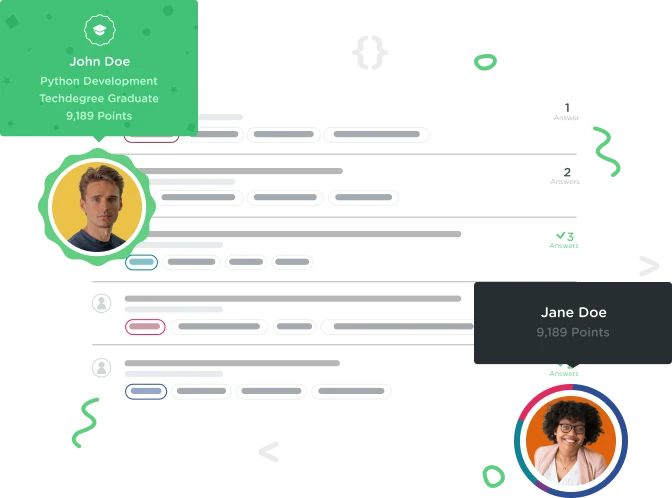
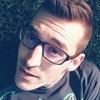
Martijn Brackman
Full Stack JavaScript Techdegree Student 3,721 PointsMade a Quiz! Can someone review my code?
function print(message) {
document.write(message);
}
// Array with questions and answers
var quizQuestions = [
["Wat is mijn voornaam?","martijn"],
["Wat is mijn achternaam?","brackman"],
["Wat is de naam van mijn bedrijf?","skroll"]
];
var userAnswer; // Storing answer
var correctAnswer = []; // Array to store whether the answer was correct or not.
var numberCorrectAnswers = 0; // Counter
var correctList = ""; // Storing all correct questions.
var wrongList = ""; // Storing all wrong questions.
var html; // Storing message output.
// Ask and verify the questions
for (var i = 0; i < quizQuestions.length; i += 1) {
userAnswer = prompt (quizQuestions [i][0]);
if (userAnswer.toLowerCase() === quizQuestions [i][1]) {
correctAnswer.push (true);
} else {
correctAnswer.push (false);
}
}
// Putting together list with correct and wrong answers
for (var i = 0; i < correctAnswer.length; i += 1) {
if (correctAnswer [i] === false) {
wrongList += "<li>" + quizQuestions [i][0] + "</li>";
} else {
correctList += "<li>" + quizQuestions [i][0] + "</li>";
numberCorrectAnswers += 1;
}
}
// Putting together message
html = "<p>You got " + numberCorrectAnswers + " question(s) right.</p><h2>You got these questions correct:</h2><ol>" + correctList + "</ol><h2>You got these questions wrong:</h2><ol>" + wrongList + "</ol>";
// Calling print function
print (html);
4 Answers
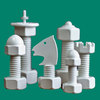
Steven Parker
229,788 PointsExcellent job! (Though I have no idea what these questions or answers are saying!)
I would make two very minor suggestions:
Replace this code:
if (userAnswer.toLowerCase() === quizQuestions [i][1]) {
correctAnswer.push (true);
} else {
correctAnswer.push (false);
}
...with this line:
correctAnswer [i] = userAnswer.toLowerCase() === quizQuestions [i][1];
This not only makes the code a bit more compact, but the use of subscripting is consistent with the way the list is handled later. Mixing "push" with subscripts can potentially cause trouble if future code changes are made.
My other suggestion is to replace this very long line:
var html = "<p>You got " + numberCorrectAnswers + " question(s) right.</p><h2>You got these questions correct:</h2><ol>" + correctList + "</ol><h2>You got these questions wrong:</h2><ol>" + wrongList + "</ol>";
...with these lines:
var html = "<p>You got " + numberCorrectAnswers
+ " question(s) right.</p><h2>You got these questions correct:</h2><ol>"
+ correctList
+ "</ol><h2>You got these questions wrong:</h2><ol>"
+ wrongList + "</ol>";
And that's simply to enhance readability.
Keep up the good work, and happy coding! -sp

Thomas Nilsen
14,957 PointsI agree - great work! :)
I have some suggestions though (I'll post the code at the end this);
- You have a 2D array where index 0 is question, and 1 is answer. I would recommend changing the structure to an object
- Make functions for repetitive tasks. Right now you have one function that writes the output, once. No need for a function for that.
- You can easily avoid the if/else in your first loop, and write it much cleaner (see code below).
- When declaring multiple variables, you don't need 'var' on every line, just comma-separate them (this is just personal preference)
- You have two loops, one where you store the correct/false answers, and one for making the correct/wrong lists. This could be done in one loop, I don't include this in my code below though
var quizQuestions = [
{ question: 'Wat is mijn voornaam?', answer: 'martijn' },
{ question: 'Wat is mijn achternaam?', answer: 'brackman' },
{ question: 'Wat is de naam van mijn bedrijf?', answer: 'skroll' }
];
var correctAnswer = [], // Array to store whether the answer was correct or not.
correctList = "", // Storing all correct questions.
wrongList = ""; // Storing all wrong questions.
quizQuestions.forEach(function(obj) {
var userAnswer = prompt(obj.question),
result = userAnswer.toLowerCase() === obj.answer; //This will evaluate to either true or false, which means you can just store the result
correctAnswer.push(result);
});
correctAnswer.forEach(function(answer, i) {
if(answer === false) wrongList += "<li>" + quizQuestions[i].question + "</li>";
else correctList += "<li>" + quizQuestions[i].question + "</li>";
});
// Putting together message
var html = "<p>You got " + correctList.length + " question(s) right.</p><h2>You got these questions correct:</h2><ol>" + correctList + "</ol><h2>You got these questions wrong:</h2><ol>" + wrongList + "</ol>";
// Printing the result
document.write(html);
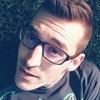
Martijn Brackman
Full Stack JavaScript Techdegree Student 3,721 PointsHi Steven
Just te be sure about this line of code:
correctAnswer [i] = userAnswer.toLowerCase() === quizQuestions [i][1];
Is it correct when I say Javascript automatically sees this as true or false? And is "true" or "false" what is pushed to my array? So it is correct to assume that I get the same result as I was trying to achieve with "correctAnswer.push"?
I only started learning JavaScript a couple of days ago so I have a lot to find out. Thank you very much for the reply.
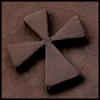
Mindaugas Krivickas
9,853 PointsHere is my code: https://w.trhou.se/9ui822ziu2
Martijn Brackman
Full Stack JavaScript Techdegree Student 3,721 PointsMartijn Brackman
Full Stack JavaScript Techdegree Student 3,721 PointsCondensed my code a tiny bit.