Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial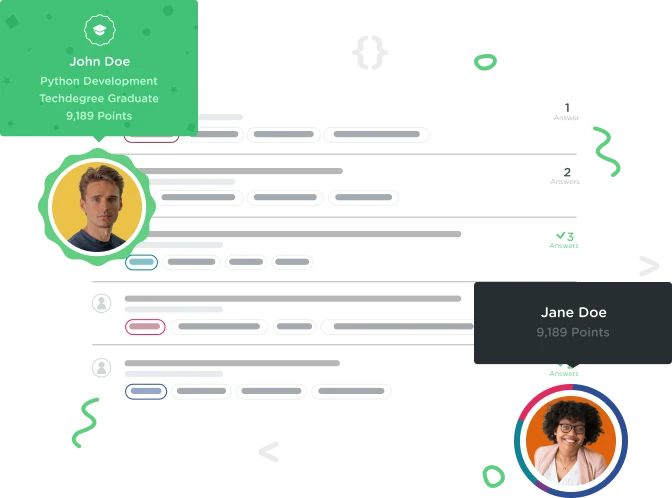

Nick Chew
5,668 PointsMade a random funny Mafia / Hitman simulation (FOR FUN)
Hi guys. Just made this random funny Mafia / Hitman simulation (FOR FUN and practice). Anyone can add in stuff if they want. LoL. I've saved it on my computer and will come back to it when I finish this course. Have fun!
var gunsBlazing;
var gunsBlazingKills = Math.floor(Math.random() * 11) + 1;
var sniperRifle;
var mafiaAssassinated = false;
var kidnappedFamily = true;
var choices = prompt("The whole Mafia gang, including the Leader and his family, gathered in a room. Do you charge in guns blazing or just use a sniper rifle like a normal person?");
/* Player chooses either guns blazing or sniper rifle options. */
if ( choices === gunsBlazing ) {
document.write("<p>You charged in guns blazing and killed " + gunsBlazingKills + "gang members!</p>");
} else if ( choices === sniperRifle ) {
mafiaAssassinated = true;
document.write("<p>You shot and killed the Mafia Leader!</p>");
}
if ( mafiaAssassinated ) {
document.write("<p>The Mafia Leader has been assassinated! Mission accomplished!</p>");
} else if ( kidnappedFamily ) {
document.write("<p>The Mafia Leader escaped but you have taken his family!</p>");
} else {
document.write("<p>Mission failed!</p>");
}
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsOkay so a couple of suggestions/comments:
gunsBlazing
and sniperRifle
are names of variables, not strings, so the value received in the prompt
will never equal either of them (they are both technically equal to undefined
, which is sort of its own type just for variables that haven't been given any value).
If you get rid of the gunsBlazing
and sniperRifle
variables, and change the first conditional statement to check for matching strings, then it can run successfully:
if ( choices === "guns blazing") {
document.write("<p>You charged in guns blazing and killed " + gunsBlazingKills + "gang members!</p>");
} else if ( choices === "sniper rifle" ) {
mafiaAssassinated = true;
document.write("<p>You shot and killed the Mafia Leader!</p>");
}
Alternatively, you could assign the variables a value and check that choices
matches it.
var gunsBlazing = "guns blazing";
var sniperRifle = "sniper rifle";
if ( choices === gunsBlazing) {
document.write("<p>You charged in guns blazing and killed " + gunsBlazingKills + "gang members!</p>");
} else if ( choices === sniperRifle ) {
mafiaAssassinated = true;
document.write("<p>You shot and killed the Mafia Leader!</p>");
}
You could even use a numbered option system, and maybe an else
clause:
var gunsBlazing = 1;
var sniperRifle = 2;
var choices = prompt("The whole Mafia gang, including the Leader and his family, gathered in a room. Do you: (1): charge in guns blazing or (2): just use a sniper rifle like a normal person?");
/* Player chooses either 1 for guns blazing or 2 for sniper rifle */
if ( parseInt(choices) === gunsBlazing) {
document.write("<p>You charged in guns blazing and killed " + gunsBlazingKills + "gang members!</p>");
} else if ( parseInt(choices) === sniperRifle ) {
mafiaAssassinated = true;
document.write("<p>You shot and killed the Mafia Leader!</p>");
} else {
kidnappedFamily = false;
document.write("<p>The Mafia goons discovered you in the bushes while you were trying to decide on a weapon...</p>");
}
Because in your original code you've set kidnappedFamily
to true
and don't give any option to change it during the game, the player would never 'fail' (that is, the final else
would never run).
One other thing you could try to add is the ability to kill the leader while going in guns blazing... maybe by getting the highest number possible from the random number generation, which in your case would be 11. You'd need to check that they chose guns blazing AND that gunsBlazingKills equals 11.
AND / OR hasn't been covered yet in the course though, so maybe come back after it has.
john larson
16,594 Pointsjohn larson
16,594 PointsI like the idea of what you did, clever. I wasn't sure (as a player) what to enter at the first prompt. It did give me a chuckle though reading through your code.